c语言结构体数组的排序方法
时间: 2023-12-18 11:30:21 浏览: 51
C语言结构体数组的排序方法可以使用标准库函数`qsort()`来实现。`qsort()`函数可以对任意类型的数组进行排序,包括结构体数组。
下面是一个示例代码,演示了如何使用`qsort()`函数对结构体数组按照某个字段进行排序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义一个学生结构体
struct student {
char name[20];
int age;
char course[20];
int score;
};
// 比较函数,用于指定排序规则
int compare(const void *a, const void *b) {
// 将void指针转换为结构体指针
const struct student *s1 = (const struct student *)a;
const struct student *s2 = (const struct student *)b;
// 按照分数降序排序
if (s1->score < s2->score) {
return 1;
} else if (s1->score > s2->score) {
return -1;
} else {
return 0;
}
}
int main() {
// 定义一个学生结构体数组
struct student st[3] = {
{ "周",50, "java", 90 },
{ "翟", 25, "C++", 97 },
{ "催", 25, "python", 92 }
};
// 使用qsort函数对结构体数组进行排序
qsort(st, 3, sizeof(struct student), compare);
// 打印排序后的结果
for (int i = 0; i < 3; i++) {
printf("姓名:%s,年龄:%d,课程:%s,分数:%d\n", st[i].name, st[i].age, st[i].course, st[i].score);
}
return 0;
}
```
运行以上代码,将会按照分数的降序对结构体数组进行排序,并输出排序后的结果。
相关推荐
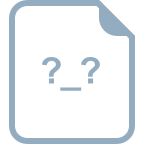
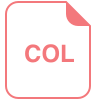
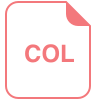
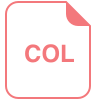
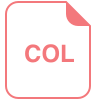









