创建单链表时需带有头结点,既可以顺序构建也可以逆序构建,但单链表的长度和其中的结点元素必须是任意输入的。 2.对创建的两个单链表和归并后的单链表在主函数中分别进行输出。
时间: 2024-10-27 10:07:15 浏览: 17
创建单链表时,确实需要一个头结点作为起点,无论是顺序还是逆序插入节点,都是从头结点开始操作。首先,你可以定义一个结构体或类`ListNode`,包含一个数据域`data`和一个指向下一个节点的指针`next`。
对于顺序构建,你通常会有一个循环或递归过程,初始化头结点,然后依次添加新节点到链表的尾部。例如:
```cpp
ListNode* buildSequentialList(int* input, int length) {
ListNode head;
head.data = input[0];
ListNode* current = &head;
for (int i = 1; i < length; i++) {
ListNode newNode;
newNode.data = input[i];
current->next = &newNode;
current = &newNode;
}
return head;
}
对于逆序构建,你会从列表的最后一个元素开始向前添加:
```cpp
ListNode* buildReversedList(int* input, int length) {
ListNode* tail = new ListNode{input[length - 1]};
ListNode* current = tail;
for (int i = length - 2; i >= 0; i--) {
ListNode newNode;
newNode.data = input[i];
newNode.next = current->next;
current->next = &newNode;
current = &newNode;
}
return tail;
}
```
在`main`函数中,你可以通过遍历链表的方式输出每个节点的数据:
```cpp
void printList(ListNode* node) {
while (node != nullptr) {
cout << node->data << " ";
node = node->next;
}
cout << endl;
}
// 输出顺序链表和逆序链表
printList(buildSequentialList(inputArray, listLength));
printList(buildReversedList(inputArray, listLength));
```
阅读全文
相关推荐
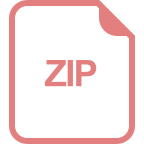
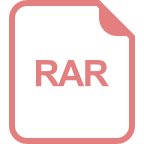
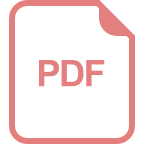


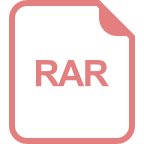
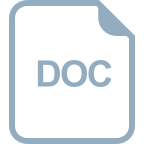
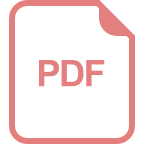
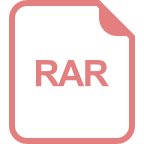
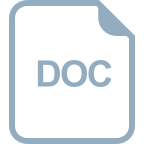
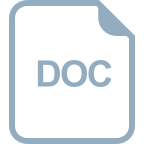






