C语言编程实现: 1、正序生成单链表或者逆序生成单链表; 2、在生成的单链表中插入一个元素结点; 3、在单链表中删除一个元素结点并回收该结点。
时间: 2023-05-22 09:02:47 浏览: 90
对于第一个问题,实现正序或逆序生成单链表的代码示例如下:
// 定义单链表结构体
struct ListNode {
int val;
struct ListNode *next;
};
// 正序生成单链表
struct ListNode* createList(int* nums, int numsSize) {
struct ListNode* head = NULL;
struct ListNode* tail = NULL;
for (int i = 0; i < numsSize; i++) {
struct ListNode* node = malloc(sizeof(struct ListNode));
node->val = nums[i];
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
// 逆序生成单链表
struct ListNode* createListReverse(int* nums, int numsSize) {
struct ListNode* head = NULL;
for (int i = numsSize - 1; i >= 0; i--) {
struct ListNode* node = malloc(sizeof(struct ListNode));
node->val = nums[i];
node->next = head;
head = node;
}
return head;
}
对于第二个问题,在单链表中插入一个元素结点的代码示例如下:
// 在单链表中插入一个元素结点
void insertNode(struct ListNode* head, int index, int val) {
struct ListNode* node = malloc(sizeof(struct ListNode));
node->val = val;
struct ListNode* prev = NULL;
struct ListNode* curr = head;
for (int i = 0; i < index && curr != NULL; i++) {
prev = curr;
curr = curr->next;
}
if (prev == NULL) {
node->next = head;
head = node;
} else {
prev->next = node;
node->next = curr;
}
}
对于第三个问题,在单链表中删除一个元素结点并回收该结点的代码示例如下:
// 在单链表中删除一个元素结点并回收该结点
void deleteNode(struct ListNode* head, int index) {
struct ListNode* prev = NULL;
struct ListNode* curr = head;
for (int i = 0; i < index && curr != NULL; i++) {
prev = curr;
curr = curr->next;
}
if (prev == NULL) {
head = curr->next;
} else {
prev->next = curr->next;
}
free(curr);
}
阅读全文
相关推荐
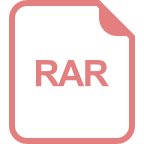
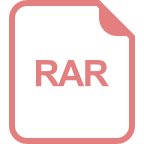
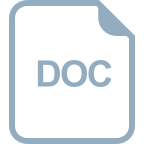


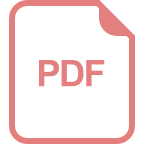
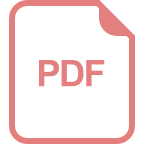
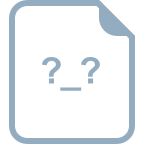
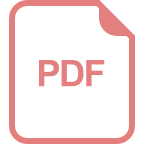
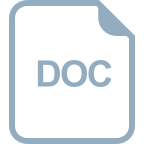






