输入N个单词,建立单链表,逆序存储这些单词,然后从头结点开始遍历输出,(递归实现)C语言
时间: 2023-08-22 08:06:43 浏览: 100
以下是一个递归实现的C程序,可以将输入的N个单词建立单链表,逆序存储,然后从头结点开始遍历输出:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct node {
char word[50];
struct node *next;
} Node;
Node *createList(int n);
void printList(Node *head);
int main() {
int n;
printf("请输入单词个数:");
scanf("%d", &n);
Node *head = createList(n);
printf("逆序存储的单词为:");
printList(head);
return 0;
}
Node *createList(int n) {
if (n <= 0) {
return NULL;
}
Node *head = (Node *)malloc(sizeof(Node));
printf("请输入单词:");
scanf("%s", head->word);
head->next = createList(n - 1);
return head;
}
void printList(Node *head) {
if (head == NULL) {
return;
}
printList(head->next);
printf("%s ", head->word);
}
```
程序中,首先输入单词个数N,然后调用createList()函数创建单链表。createList()函数递归地创建单链表,每次输入一个单词,然后将其插入到链表的头部。最终,函数返回头结点的指针。
接着,调用printList()函数遍历输出单链表。printList()函数也是递归实现的,每次先遍历链表的后继节点,再输出当前节点的单词。这样就可以实现逆序输出单链表。
阅读全文
相关推荐
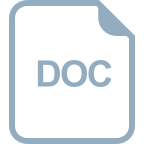
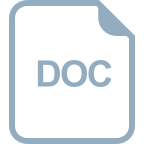
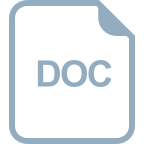



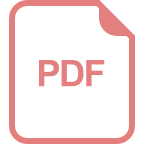











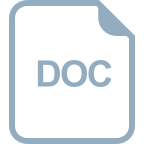