编写一个C程序,输入N个单词,建一个单链表,然后从头节点开始遍历输出,递归实现。例如输入3 one two three输出Three two one
时间: 2024-03-20 21:44:10 浏览: 60
好的,下面是C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义单链表结构体
struct node {
char word[20];
struct node *next;
};
// 递归遍历链表并输出
void printList(struct node *head) {
if (head == NULL) {
return;
}
printList(head->next);
printf("%s ", head->word);
}
int main() {
int n, i;
char w[20];
struct node *head, *p, *q;
printf("请输入单词的数量:");
scanf("%d", &n);
// 建立单链表
head = NULL;
for (i = 0; i < n; i++) {
printf("请输入第%d个单词:", i + 1);
scanf("%s", w);
p = (struct node *)malloc(sizeof(struct node));
strcpy(p->word, w);
p->next = NULL;
if (head == NULL) {
head = p;
} else {
q->next = p;
}
q = p;
}
// 输出单链表
printf("单链表输出结果:");
printList(head);
printf("\n");
return 0;
}
```
输入N个单词后,程序会逐个创建单链表节点,并将节点串联起来。最后调用 `printList` 函数,以递归方式遍历链表并输出单词。注意,这里借助了递归函数的特性:当链表到达末尾时,才会开始输出单词,因此输出的顺序是逆序的。
阅读全文
相关推荐
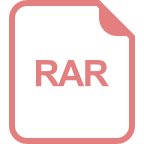






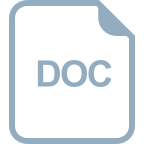
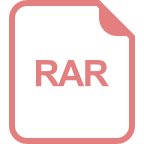
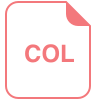
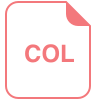




