通用 任意数组 一个含有字符串的数组,例如:["a","b","a","c","b","d","a"],需要得到一个新数组 ["a1","b1","a2","c","b2","d","a3"] 要求: 未重复的元素不处理 重复的元素加上编号 不能影响正常数组的顺序
时间: 2024-06-02 21:04:40 浏览: 13
通用的任意数组是指在定义时不限定数组元素的类型和个数的数组。在C++中,可以使用模板来定义通用的任意数组。
对于题目中提到的需求,可以通过遍历原数组,使用一个哈希表记录每个元素出现的次数,并在遍历过程中生成新的数组,对于重复的元素,在其后面添加编号即可。
以下是示例代码实现:
```c++
#include <iostream>
#include <unordered_map>
#include <vector>
#include <string>
using namespace std;
vector<string> processArray(vector<string> &arr) {
unordered_map<string, int> freq; // 哈希表记录每个元素出现次数
vector<string> res; // 新数组
for (auto &s : arr) {
if (freq.count(s) == 0) { // 未出现过的元素直接添加到新数组中
res.push_back(s);
freq[s] = 1;
} else { // 出现过的元素添加编号后加入新数组
int cnt = freq[s];
res.push_back(s + to_string(cnt));
freq[s] = cnt + 1;
}
}
return res;
}
int main() {
vector<string> arr = {"a", "b", "a", "c", "b", "d", "a"};
vector<string> res = processArray(arr);
for (auto &s : res) {
cout << s << " ";
}
cout << endl;
return 0;
}
```
相关推荐
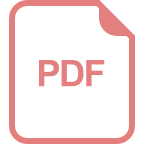
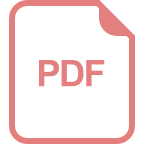
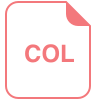













