创建maven自定义打包插件插件⽣成的zip包路径位于项⽬根⽬录下的target⽂件夹,必须基于maven⽣命中周期的compile阶段⽣成的target/classes才能完成⼯作,而且zip⽂件中必须包含可执⾏的jar包,以及程序运⾏依赖的第三jar包lib⽬录
时间: 2024-04-12 22:32:38 浏览: 21
要创建一个 Maven 自定义打包插件,可以使用 `maven-plugin-plugin` 插件来生成插件的骨架。然后在插件的逻辑中,您可以使用 `maven-assembly-plugin` 插件来完成生成 zip 包的任务。以下是实现此功能的步骤:
1. 在项目的 `pom.xml` 文件中添加 `maven-plugin-plugin` 插件的配置:
```xml
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-plugin-plugin</artifactId>
<version>3.6.0</version>
<executions>
<execution>
<id>default-descriptor</id>
<goals>
<goal>descriptor</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
```
2. 在命令行中执行以下 Maven 命令来生成插件骨架:
```shell
mvn plugin:descriptor
```
这将生成 `target/generated-sources/plugin` 目录,其中包含插件的骨架代码。
3. 在生成的插件骨架代码中,修改 `execute` 方法的逻辑以完成生成 zip 包的任务。可以参考以下示例代码:
```java
@Mojo(name = "my-zip", defaultPhase = LifecyclePhase.COMPILE)
public class MyZipMojo extends AbstractMojo {
@Parameter(defaultValue = "${project.build.outputDirectory}", required = true)
private File outputDirectory;
@Override
public void execute() throws MojoExecutionException {
try {
// 创建目标文件夹
File targetDirectory = new File(outputDirectory.getParentFile(), "target");
targetDirectory.mkdirs();
// 创建 lib 目录并复制依赖的第三方 jar 包
File libDirectory = new File(targetDirectory, "lib");
libDirectory.mkdirs();
// 从 Maven 项目中复制依赖的第三方 jar 包到 lib 目录
// 复制可执行的 jar 包到目标文件夹
File jarFile = new File(outputDirectory, "myapp.jar");
File targetJarFile = new File(targetDirectory, "myapp.jar");
Files.copy(jarFile.toPath(), targetJarFile.toPath(), StandardCopyOption.REPLACE_EXISTING);
// 创建 zip 包
File zipFile = new File(targetDirectory, "myapp.zip");
try (ZipOutputStream zos = new ZipOutputStream(new FileOutputStream(zipFile))) {
addFileToZip(targetJarFile, zos);
addFolderToZip(libDirectory, zos);
}
getLog().info("生成的 zip 包路径:" + zipFile.getAbsolutePath());
} catch (IOException e) {
throw new MojoExecutionException("无法生成 zip 包", e);
}
}
private void addFileToZip(File file, ZipOutputStream zos) throws IOException {
FileInputStream fis = new FileInputStream(file);
String entryName = file.getName();
ZipEntry zipEntry = new ZipEntry(entryName);
zos.putNextEntry(zipEntry);
byte[] buffer = new byte[1024];
int length;
while ((length = fis.read(buffer)) > 0) {
zos.write(buffer, 0, length);
}
fis.close();
zos.closeEntry();
}
private void addFolderToZip(File folder, ZipOutputStream zos) throws IOException {
for (File file : folder.listFiles()) {
if (file.isFile()) {
addFileToZip(file, zos);
} else if (file.isDirectory()) {
addFolderToZip(file, zos);
}
}
}
}
```
请注意,上面的示例代码假设您的项目使用 Maven 构建,并且您已经在项目的 `pom.xml` 文件中配置了 `maven-assembly-plugin` 插件来生成可执行的 jar 文件和依赖的第三方 jar 文件。如果您还没有配置该插件,请参考之前的回答来进行配置。
4. 使用 `mvn clean compile` 命令来编译插件,并使用 `mvn myplugin:my-zip` 命令来执行插件,生成 zip 包。
```shell
mvn clean compile
mvn myplugin:my-zip
```
这将在项目根目录下的 `target` 文件夹中生成一个名为 `myapp.zip` 的压缩包,其中包含可执行的 `myapp.jar` 文件和 `lib` 目录。
希望这可以满足您的需求!如果您有任何其他问题,请随时提问。
相关推荐
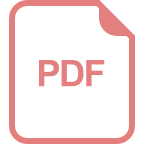
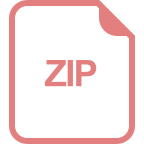
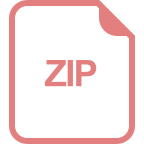














