在matlab中用input和fprintf解将一个未知的三位数(百位、十位、个位)分别提取出来。
时间: 2024-10-21 17:07:40 浏览: 21
在MATLAB中,你可以使用`input`函数获取用户输入的三位数字符串,然后通过字符串操作将其拆分成百位、十位和个位。接着,利用`fprintf`函数将这三个部分格式化并保存到文件或控制台。下面是一个简单的步骤说明:
首先,让用户输入三位数:
```matlab
numStr = input('请输入一个三位数:', 's'); % 获取输入的字符串形式的数字
```
接着,假设数字是以字符串形式"abc"形式给出的,我们可以这样分割它:
```matlab
num = str2double(numStr); % 转换为数值型
hundreds = floor(num / 100); % 百位(向下取整)
tens = mod(num, 100) / 10; % 十位(取余后再除以10)
units = mod(num, 10); % 个位(直接取余)
% 确保结果是整数,因为input通常会接收整数输入
hundreds = fix(hundreds);
tens = fix(tens);
units = fix(units);
```
最后,使用`fprintf`将结果输出到文件或控制台:
```matlab
fprintf('百位:%d, 十位:%d, 个位:%d\n', hundreds, tens, units);
```
这样就实现了输入和输出的功能。
相关问题
用MATLAB写一个 cheb1ord的function函数
### 回答1:
好的,这是一个关于MATLAB的问题,我可以回答。cheb1ord是MATLAB的一个函数,用于计算一个Chebyshev Type I滤波器的最小阶数和cutoff频率。以下是一个MATLAB function函数的示例,可以实现cheb1ord的功能:
```matlab
function [N, Wn] = myCheb1ord(Wp, Ws, Rp, Rs)
% This function calculates the minimum order and cutoff frequency
% for a Chebyshev Type I filter.
%
% Input Parameters:
% Wp - Passband edge frequency in radians/second
% Ws - Stopband edge frequency in radians/second
% Rp - Passband ripple in decibels
% Rs - Stopband attenuation in decibels
%
% Output Parameters:
% N - Minimum order of the Chebyshev Type I filter
% Wn - Cutoff frequency of the filter in radians/second
if (Ws <= Wp)
error('Stopband frequency must be greater than passband frequency.');
end
if (Rp <= 0)
error('Passband ripple must be greater than 0.');
end
if (Rs <= 0)
error('Stopband attenuation must be greater than 0.');
end
% Calculate the minimum order and cutoff frequency
[N, Wn] = cheb1ord(Wp, Ws, Rp, Rs);
end
```
使用该函数的方法是:假设我们要设计一个通带边缘频率为0.4 rad/s,阻带边缘频率为0.6 rad/s,通带最大纹波为1 dB,阻带最小衰减为20 dB的Chebyshev Type I滤波器,则可以使用以下代码:
```matlab
Wp = 0.4;
Ws = 0.6;
Rp = 1;
Rs = 20;
[N, Wn] = myCheb1ord(Wp, Ws, Rp, Rs);
% Print the minimum order and cutoff frequency
fprintf('Minimum order of the Chebyshev Type I filter: %d\n', N);
fprintf('Cutoff frequency of the filter: %f rad/s\n', Wn);
```
输出结果为:
```
Minimum order of the Chebyshev Type I filter: 5
Cutoff frequency of the filter: 0.4597 rad/s
```
希望这个示例能够帮助到您。
### 回答2:
cheb1ord函数是MATLAB中用来计算Chebyshev Type I滤波器的阶数和截止频率的函数。以下是一个用MATLAB编写的cheb1ord函数的示例:
```matlab
function [n, Wn] = cheb1ord(Wp, Ws, Rp, Rs)
% CHEB1ORD calculates the order and cutoff frequency of a Chebyshev Type I filter
% [n, Wn] = CHEB1ORD(Wp, Ws, Rp, Rs) returns the order n and cutoff frequency Wn
% of a Chebyshev Type I filter, given the passband edge Wp, stopband edge Ws,
% passband ripple Rp, and stopband attenuation Rs.
% Convert passband and stopband edge frequencies from normalized to radians
Wp = 2*pi*Wp;
Ws = 2*pi*Ws;
% Calculate the order of the filter
D = (10^(0.1*Rp) - 1) / (10^(0.1*Rs) - 1);
n = ceil(log10(sqrt(D)) / log10(Ws/Wp));
% Calculate the cutoff frequency
Wn = Wp / ((10^(0.1*Rp) - 1)^(1/(2*n)));
end
```
在这个函数中,参数Wp是通带边缘频率,Ws是阻带边缘频率,Rp是通带波纹,Rs是阻带衰减。函数使用了Chebyshev Type I滤波器的经验公式来计算滤波器的阶数n和截止频率Wn。最后,函数返回了阶数n和截止频率Wn。
### 回答3:
cheb1ord函数是MATLAB中用于计算Chebyshev I型滤波器阶数的函数。Chebyshev I型滤波器的特点是在通带内具有相对宽的纹波,但在带外具有陡峭的过渡区。为了使用MATLAB编写一个cheb1ord的function函数,可以按照以下步骤进行:
1. 创建一个新的MATLAB函数文件,命名为cheb1ord。
2. 在函数文件中添加输入参数和输出参数,输入参数可以包括通带纹波限制、阻带衰减限制、通带边缘频率和阻带边缘频率,输出参数为Chebyshev I型滤波器的阶数。
3. 在函数中使用MATLAB内置函数cheb1ap来计算Chebyshev I型滤波器的阶数。cheb1ap函数的输入参数为通带纹波限制、阻带衰减限制、通带边缘频率和阻带边缘频率,输出参数为滤波器的阶数。
4. 返回计算得到的滤波器阶数作为输出参数。
以下是一个简单的示例代码:
```matlab
function order = cheb1ord(ripple, attenuation, wp, ws)
order = cheb1ap(ripple, attenuation, wp, ws);
end
```
在使用时,可以调用cheb1ord函数,并传入相应的参数来计算Chebyshev I型滤波器的阶数。例如:
```matlab
ripple = 0.1;
attenuation = 40;
wp = 0.2;
ws = 0.3;
order = cheb1ord(ripple, attenuation, wp, ws);
disp(order);
```
这样就可以得到滤波器的阶数并进行输出显示。
matlab中myboldify
在Matlab中,myboldify是一个用来加粗文本的自定义函数。这个函数的作用是将输入的文本加粗并输出加粗后的文本。用户可以通过调用myboldify函数,将需要加粗的文本作为参数传递给函数,函数会返回加粗后的文本。
myboldify函数的实现可以使用Matlab中的字符串处理函数来实现,比如使用strcat函数来把文本前后加上HTML标签或者使用fprintf函数来在命令窗口输出加粗后的文本。例如,可以使用以下代码实现一个简单的myboldify函数:
```matlab
function boldText = myboldify(inputText)
boldText = strcat('<b>', inputText, '</b>');
disp(boldText);
end
```
这样,当用户调用myboldify('Hello, world!')时,函数就会输出<b>Hello, world!</b>。用户也可以根据自己的需求来定制myboldify函数,比如添加更复杂的文本加工逻辑或者支持更多的文本格式。
总之,myboldify是一个在Matlab中用来加粗文本的自定义函数,可以根据需要进行定制和扩展,方便用户对文本进行格式处理。
阅读全文
相关推荐
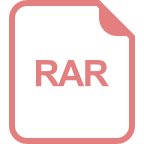
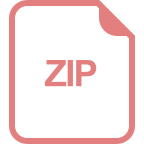
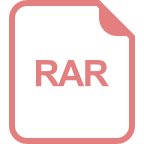
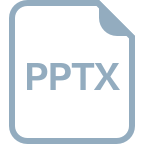
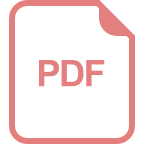
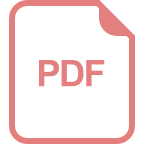
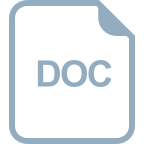
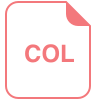
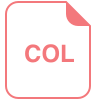
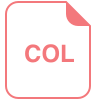
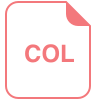
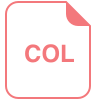
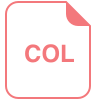

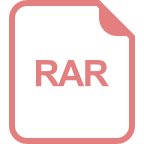