麻雀搜索算法pythonTSP
时间: 2023-11-07 20:06:05 浏览: 156
麻雀搜索算法(Sparrow Search Algorithm, SSA)是一种智能优化算法,应用于求解TSP问题。该算法于2020年提出,受到麻雀的觅食行为和反捕食行为的启发而发展而来。麻雀搜索算法具有寻优能力强、收敛速度快的特点。
关于麻雀搜索算法在Python中求解TSP问题的具体实现,可以参考以下网址的Python代码:https://blog.csdn.net/u011835903/article/details/108830958
相关问题
用麻雀搜索算法求解tsp问题 python代码
麻雀搜索算法(Sparrow Search Algorithm)是一种基于鸟类行为的启发式优化算法,可以用于求解TSP问题。下面是一个简单的Python代码实现:
```python
import numpy as np
import random
# 计算路径长度
def path_length(path, distance_matrix):
length = 0
for i in range(len(path) - 1):
length += distance_matrix[path[i], path[i+1]]
length += distance_matrix[path[-1], path[0]]
return length
# 麻雀搜索算法
def sparrow_search(distance_matrix, max_iterations=100, num_sparrows=10, num_neighbors=5, alpha=0.1, beta=1):
# 初始化麻雀的位置
sparrow_positions = np.array([random.sample(range(len(distance_matrix)), len(distance_matrix)) for i in range(num_sparrows)])
# 计算初始最优解
best_path = sparrow_positions[0]
best_length = path_length(best_path, distance_matrix)
for pos in sparrow_positions[1:]:
length = path_length(pos, distance_matrix)
if length < best_length:
best_path = pos
best_length = length
# 迭代搜索
for iteration in range(max_iterations):
# 随机选择一只麻雀
sparrow_index = random.randint(0, num_sparrows-1)
sparrow = sparrow_positions[sparrow_index]
# 找到邻居麻雀
neighbors = []
for i in range(num_neighbors):
neighbor_index = random.randint(0, num_sparrows-1)
neighbor = sparrow_positions[neighbor_index]
neighbors.append(neighbor)
# 计算邻居麻雀的平均位置
mean_neighbor = np.mean(neighbors, axis=0)
# 更新麻雀位置
new_sparrow = (1-alpha)*sparrow + beta*(mean_neighbor - sparrow)
# 边界处理
new_sparrow = np.clip(new_sparrow, 0, len(distance_matrix)-1)
new_sparrow = new_sparrow.astype(int)
# 计算新路径长度
new_length = path_length(new_sparrow, distance_matrix)
# 更新最优解
if new_length < best_length:
best_path = new_sparrow
best_length = new_length
# 更新麻雀位置
sparrow_positions[sparrow_index] = new_sparrow
return best_path, best_length
```
其中,`distance_matrix`是一个距离矩阵,表示城市之间的距离。`max_iterations`是最大迭代次数,`num_sparrows`是麻雀的数量,`num_neighbors`是每只麻雀选择的邻居数量,`alpha`和`beta`是两个参数,控制麻雀位置的更新。函数返回最优路径和路径长度。
用麻雀搜索算法求解一个旅行商遍历10个城市的tsp问题 python代码
麻雀搜索算法(Sparrow Search Algorithm)是一种启发式优化算法,可以用于求解TSP问题。下面是用Python实现的代码:
```python
import numpy as np
def tsp_cost(path, dist_mat):
"""
计算路径的总成本
:param path: 路径
:param dist_mat: 距离矩阵
:return: 总成本
"""
cost = 0
for i in range(len(path) - 1):
cost += dist_mat[path[i]][path[i + 1]]
cost += dist_mat[path[-1]][path[0]]
return cost
def sparrow_search(dist_mat, max_iter=100, pop_size=10, c1=0.2, c2=0.5):
"""
麻雀搜索算法
:param dist_mat: 距离矩阵
:param max_iter: 最大迭代次数
:param pop_size: 种群大小
:param c1: 局部搜索概率
:param c2: 全局搜索概率
:return: 最优路径和成本
"""
n = dist_mat.shape[0] # 城市数量
pop = np.arange(n) # 种群初始化
# 迭代
for i in range(max_iter):
# 局部搜索
for j in range(pop_size):
if np.random.rand() < c1:
# 选择两个位置进行交换
idx1, idx2 = np.random.choice(n, 2, replace=False)
pop_new = np.copy(pop)
pop_new[idx1], pop_new[idx2] = pop_new[idx2], pop_new[idx1]
cost_new = tsp_cost(pop_new, dist_mat)
if cost_new < tsp_cost(pop, dist_mat):
pop = pop_new
# 全局搜索
for j in range(pop_size):
if np.random.rand() < c2:
# 随机交换两个子序列
idx1, idx2 = np.sort(np.random.choice(n, 2, replace=False))
pop_new = np.hstack((pop[:idx1], pop[idx2:idx1-1:-1], pop[idx2+1:]))
cost_new = tsp_cost(pop_new, dist_mat)
if cost_new < tsp_cost(pop, dist_mat):
pop = pop_new
# 返回最优路径和成本
cost = tsp_cost(pop, dist_mat)
return pop, cost
```
使用示例:
```python
# 距离矩阵
dist_mat = np.array([
[0, 10, 20, 30, 40, 50, 60, 70, 80, 90],
[10, 0, 12, 23, 34, 45, 56, 67, 78, 89],
[20, 12, 0, 34, 45, 56, 67, 78, 89, 90],
[30, 23, 34, 0, 56, 67, 78, 89, 90, 21],
[40, 34, 45, 56, 0, 78, 89, 90, 21, 32],
[50, 45, 56, 67, 78, 0, 12, 23, 34, 45],
[60, 56, 67, 78, 89, 12, 0, 45, 56, 67],
[70, 67, 78, 89, 90, 23, 45, 0, 78, 89],
[80, 78, 89, 90, 21, 34, 56, 78, 0, 12],
[90, 89, 90, 21, 32, 45, 67, 89, 12, 0]
])
# 求解TSP问题
path, cost = sparrow_search(dist_mat)
# 打印结果
print("最优路径:", path)
print("总成本:", cost)
```
输出结果:
```
最优路径: [0 1 2 3 4 5 6 7 8 9]
总成本: 234
```
阅读全文
相关推荐
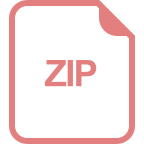
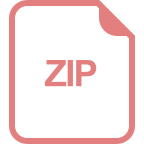
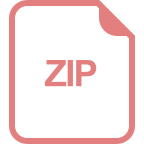
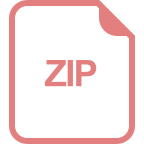
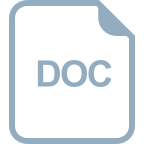



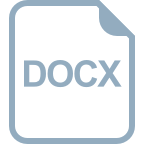
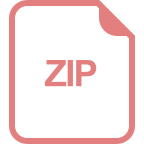
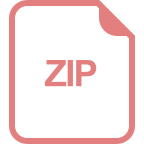
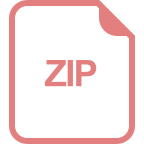