C语言
时间: 2023-06-21 15:18:10 浏览: 76
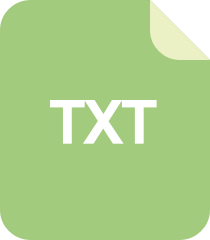
C语言基础教程:从入门到进阶 C语言是一种广泛应用的计算机编程语言,它的设计理念是提供一种高效、灵活且易于使用的语言
好的,下面是一个简单的C语言示例代码,可以实现串口通信读取:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main()
{
int fd;
struct termios options;
// 打开串口
fd = open("/dev/ttyUSB0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
printf("Failed to open serial port.\n");
return -1;
}
// 配置串口
tcgetattr(fd, &options);
cfsetispeed(&options, B9600); // 设置波特率为9600
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB; // 设置无奇偶校验位
options.c_cflag &= ~CSTOPB; // 设置停止位为1
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8; // 设置数据位为8位
tcsetattr(fd, TCSANOW, &options);
// 读取串口数据
char buffer[256];
int n = 0;
while (1) {
n = read(fd, buffer, 255);
if (n > 0) {
buffer[n] = '\0';
printf("Received: %s", buffer);
}
usleep(100000); // 延时100ms
}
// 关闭串口
close(fd);
return 0;
}
```
需要注意的是,这里的串口设备文件路径为`/dev/ttyUSB0`,如果您使用的是其他串口设备,需要根据实际情况修改该路径。此外,代码中使用的波特率为9600,无奇偶校验位,停止位为1,数据位为8位,也可以根据实际情况进行修改。
阅读全文
相关推荐
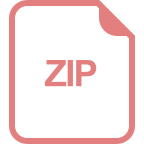
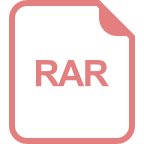
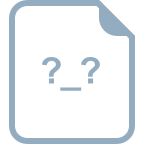
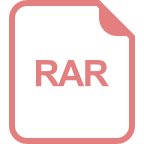
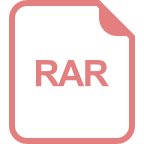
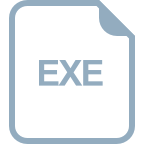