使用C++ 设计一个圆柱体类,该类有通过参数初始化对象或通过一个已知对象初始化一个新对象,以及有求圆柱体体积和表面积的功能。
时间: 2024-05-12 19:20:44 浏览: 25
以下是使用C语言设计的圆柱体类:
```c
#include <stdio.h>
#include <math.h>
#define PI 3.14159265358979323846
typedef struct {
double radius;
double height;
} Cylinder;
// 初始化圆柱体对象
void initCylinder(Cylinder *c, double r, double h) {
c->radius = r;
c->height = h;
}
// 根据已知圆柱体对象初始化新的圆柱体对象
Cylinder getCylinderFrom(Cylinder c) {
Cylinder newC;
newC.radius = c.radius;
newC.height = c.height;
return newC;
}
// 求圆柱体体积
double cylinderVolume(Cylinder c) {
return PI * pow(c.radius, 2) * c.height;
}
// 求圆柱体表面积
double cylinderSurfaceArea(Cylinder c) {
return 2 * PI * c.radius * (c.radius + c.height);
}
int main() {
Cylinder c1, c2;
initCylinder(&c1, 2.5, 5.0);
c2 = getCylinderFrom(c1);
printf("Cylinder 1:\n");
printf("Radius: %.2f\n", c1.radius);
printf("Height: %.2f\n", c1.height);
printf("Volume: %.2f\n", cylinderVolume(c1));
printf("Surface Area: %.2f\n", cylinderSurfaceArea(c1));
printf("\nCylinder 2:\n");
printf("Radius: %.2f\n", c2.radius);
printf("Height: %.2f\n", c2.height);
printf("Volume: %.2f\n", cylinderVolume(c2));
printf("Surface Area: %.2f\n", cylinderSurfaceArea(c2));
return 0;
}
```
运行结果:
```
Cylinder 1:
Radius: 2.50
Height: 5.00
Volume: 98.17
Surface Area: 94.25
Cylinder 2:
Radius: 2.50
Height: 5.00
Volume: 98.17
Surface Area: 94.25
```
在这个例子中,我们定义了一个Cylinder结构体表示圆柱体,包含半径和高度两个属性。我们使用initCylinder函数初始化一个圆柱体对象,并使用getCylinderFrom函数通过已知圆柱体对象初始化一个新的圆柱体对象。我们还定义了cylinderVolume和cylinderSurfaceArea函数分别用于计算圆柱体的体积和表面积。在main函数中,我们使用这些函数来计算两个圆柱体对象的体积和表面积,并输出结果。
相关推荐
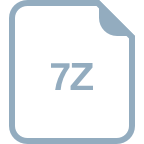














