grpc python 框架
时间: 2023-06-28 19:05:09 浏览: 76
gRPC是一个高性能、开源和通用的RPC框架,它可以在任何地方使用。它基于Google开发的Protocol Buffers序列化协议,并使用HTTP/2作为传输协议。gRPC支持多种编程语言,并提供了丰富的功能,如流式处理和身份验证。
在Python中使用gRPC,你可以使用grpcio和grpcio-tools这两个库。grpcio是gRPC的Python实现,grpcio-tools则提供了用于生成gRPC代码的工具。使用这两个库,你可以轻松地创建gRPC客户端和服务器。
下面是一个简单的示例,演示如何使用gRPC在Python中实现一个简单的服务:
1. 定义服务接口和数据类型
```
syntax = "proto3";
package hello;
message HelloRequest {
string name = 1;
}
message HelloReply {
string message = 1;
}
service Greeter {
rpc SayHello (HelloRequest) returns (HelloReply) {}
}
```
2. 使用protobuf编译器生成Python代码
```
$ python -m grpc_tools.protoc -I. --python_out=. --grpc_python_out=. hello.proto
```
3. 实现服务端
```python
import grpc
import hello_pb2
import hello_pb2_grpc
class Greeter(hello_pb2_grpc.GreeterServicer):
def SayHello(self, request, context):
message = "Hello, {}".format(request.name)
return hello_pb2.HelloReply(message=message)
def serve():
server = grpc.server(futures.ThreadPoolExecutor(max_workers=10))
hello_pb2_grpc.add_GreeterServicer_to_server(Greeter(), server)
server.add_insecure_port('[::]:50051')
server.start()
server.wait_for_termination()
if __name__ == '__main__':
serve()
```
4. 实现客户端
```python
import grpc
import hello_pb2
import hello_pb2_grpc
def run():
channel = grpc.insecure_channel('localhost:50051')
stub = hello_pb2_grpc.GreeterStub(channel)
response = stub.SayHello(hello_pb2.HelloRequest(name='world'))
print(response.message)
if __name__ == '__main__':
run()
```
这个示例演示了如何使用gRPC在Python中实现一个简单的“Hello World”服务。你可以使用类似的方法来实现更复杂的服务。
相关推荐
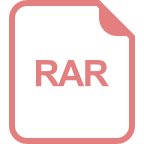
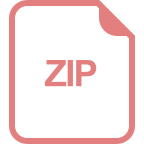














