定义一个 Retangle类,有长、宽等属性,定义构造方法用来初始化类的这些属性,定义方法输出Retangle的长宽和面积。编写应用程序使用Retangle。 【输入形式】 Please input length: Please input width: 【输出形式】 The lengh is: The width is: The area is: 【输入样例】 4.0 3.0 【输出样例】 The lengh is:4.0 The width is:3.0 The area is:12.0
时间: 2024-02-20 22:58:23 浏览: 55
下面是 Python3 的代码实现:
```python
class Rectangle:
def __init__(self, length, width):
self.length = length
self.width = width
def get_length(self):
return self.length
def get_width(self):
return self.width
def get_area(self):
return self.length * self.width
length = float(input("Please input length: "))
width = float(input("Please input width: "))
rectangle = Rectangle(length, width)
print("The lengh is:", rectangle.get_length())
print("The width is:", rectangle.get_width())
print("The area is:", rectangle.get_area())
```
相关问题
. 定义矩形类Rectangle,含长、宽属性,静态属性ID; 定义两个构造方法,一个默认,一个能初始化长宽属性; 定义求面积、求周长方法,能返回计算结果; 定义长宽属性访问方法和设置方法(称为JavaBean); 定义toString()方法,返回对象信息; 定义静态方法,获取静态属性ID; 定义静态初始化块,设置ID属性; 主类main方法中定义不同类对象,应用所有方法。
好的,以下是矩形类Rectangle的代码实现:
```java
public class Rectangle {
private double length;
private double width;
private static int ID;
public Rectangle() {
this.length = 0;
this.width = 0;
}
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
public double getLength() {
return length;
}
public void setLength(double length) {
this.length = length;
}
public double getWidth() {
return width;
}
public void setWidth(double width) {
this.width = width;
}
public double getArea() {
return length * width;
}
public double getPerimeter() {
return 2 * (length + width);
}
public static int getID() {
return ID;
}
static {
ID = 1000;
}
@Override
public String toString() {
return "Rectangle [length=" + length + ", width=" + width + "]";
}
}
```
在主类中可以应用所有方法:
```java
public class Main {
public static void main(String[] args) {
Rectangle r1 = new Rectangle();
Rectangle r2 = new Rectangle(3, 4);
r1.setLength(5);
r1.setWidth(6);
System.out.println("Rectangle r1's area is: " + r1.getArea());
System.out.println("Rectangle r1's perimeter is: " + r1.getPerimeter());
System.out.println("Rectangle r1's ID is: " + Rectangle.getID());
System.out.println("Rectangle r1's info is: " + r1.toString());
System.out.println("Rectangle r2's area is: " + r2.getArea());
System.out.println("Rectangle r2's perimeter is: " + r2.getPerimeter());
System.out.println("Rectangle r2's ID is: " + Rectangle.getID());
System.out.println("Rectangle r2's info is: " + r2.toString());
}
}
```
输出结果如下:
```
Rectangle r1's area is: 30.0
Rectangle r1's perimeter is: 22.0
Rectangle r1's ID is: 1000
Rectangle r1's info is: Rectangle [length=5.0, width=6.0]
Rectangle r2's area is: 12.0
Rectangle r2's perimeter is: 14.0
Rectangle r2's ID is: 1000
Rectangle r2's info is: Rectangle [length=3.0, width=4.0]
```
定义一个矩形类Rectangle,属性包括长和宽,在构造方法中将长宽初始化、再定义一个成员方法用于求矩形的面积 定义测试类RectangleTest测试求矩形的面积。
下面是矩形类Rectangle的定义:
```java
public class Rectangle {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
public double getArea() {
return length * width;
}
}
```
在这个类中,我们有两个私有属性:length和width,分别表示矩形的长和宽。构造方法用于初始化这两个属性。getArea方法用于计算矩形的面积并返回。
下面是测试类RectangleTest的定义:
```java
public class RectangleTest {
public static void main(String[] args) {
Rectangle rectangle = new Rectangle(3, 4);
double area = rectangle.getArea();
System.out.println("矩形的面积为:" + area);
}
}
```
在这个测试类中,我们创建了一个矩形对象并将其长和宽初始化为3和4。然后调用getArea方法计算出矩形的面积并输出。
阅读全文
相关推荐
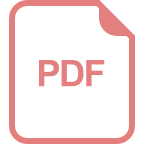
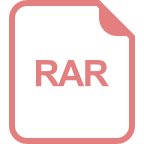
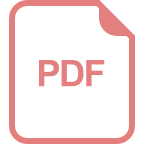













