请根据裁判程序和输入输出样例,定义一个Tree类,具体要求如下: 1.私有数据成员:树名、树龄。 2.成员函数:无参构造函数,带2个参数的构造函数(树龄的默认值为0)。 类: class Tree 裁判测试程序样例: #include <iostream> #include <string> using namespace std; /* 请在这里填写答案 */ int main() { string name; //树名 int age;//树龄 cin>>name>>age; Tree t1,t2(name),t3(name,age); return 0; } 输入样例: 白桦树 10 输出样例: 无名树 白桦树,树龄:0年 白桦树,树龄:10年
时间: 2024-03-03 16:47:57 浏览: 40
根据题目要求,`Tree` 类需要包含两个私有数据成员:`name` 和 `age`,以及两个构造函数,一个是无参构造函数,一个是带两个参数的构造函数,其中树龄的默认值为0。下面是 `Tree` 类的定义:
```c++
#include <string>
using namespace std;
class Tree {
private:
string name; // 树名
int age; // 树龄
public:
Tree() { // 无参构造函数
name = "无名树";
age = 0;
}
Tree(string name, int age = 0) { // 带两个参数的构造函数
this->name = name;
this->age = age;
}
string getName() { // 获取树名
return name;
}
int getAge() { // 获取树龄
return age;
}
};
```
在上面的代码中,我们添加了两个私有数据成员 `name` 和 `age`,并且定义了两个构造函数,一个是无参构造函数,一个是带两个参数的构造函数。另外,我们加入了两个公有函数 `getName()` 和 `getAge()`,用于获取树的名称和年龄。
在 `main()` 函数中,你可以像下面这样创建三个树对象:
```c++
int main() {
string name;
int age;
cin >> name >> age;
Tree t1;
Tree t2(name);
Tree t3(name, age);
cout << t1.getName() << endl;
cout << t2.getName() << ",树龄:" << t2.getAge() << "年" << endl;
cout << t3.getName() << ",树龄:" << t3.getAge() << "年" << endl;
return 0;
}
```
这个程序会根据输入的参数创建三个树对象,并输出它们的名称和年龄。如果输入的参数是 `白桦树 10`,那么输出结果应该是:
```
无名树
白桦树,树龄:0年
白桦树,树龄:10年
```
相关推荐
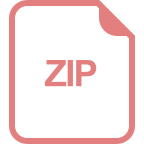
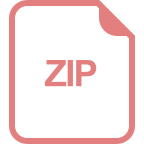











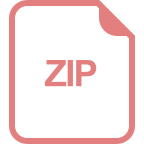
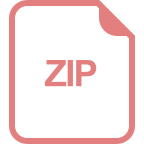
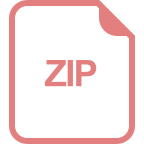
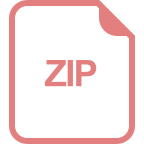