解释from PIL import Image img1=Image.open('狗.jpg').convert(mode='RGB') img2=Image.open('蜜蜂.jpg').convert(mode='RGB') img3=img2.copy() region=img3.crop((600,150,1080,650)) region1=region.resize((200,300)) img1.paste(region1,(600,600)) img1.show()
时间: 2023-05-29 12:07:50 浏览: 212
这段代码使用Python中的PIL模块(Python Imaging Library)来对两张图片进行操作。第一行导入了Image模块,用于处理图像。第二行使用Image.open打开了一张名为“狗.jpg”的图片,并将其转换为RGB模式。第三行也是使用Image.open打开了一张名为“蜜蜂.jpg”的图片,并将其转换为RGB模式。第四行使用img2.copy()创建了img3的副本。第五行使用img3.crop()方法裁剪出图片中的一个区域,该区域的左上角坐标为(600, 150),右下角坐标为(1080, 650)。第六行使用region.resize()方法将裁剪出来的区域缩放为(200, 300)的大小。第七行使用img1.paste()方法将缩放后的区域粘贴到img1中,位置为(600, 600)。最后一行使用img1.show()方法显示处理后的图片。
相关问题
from PIL import Image img1=Image.open('狗.jpg').convert(mode='RGB') img2=Image.open('蜜蜂.jpg').convert(mode='RGB') img3=img2.copy() region=img3.crop((600,150,1080,650)) region1=region.resize((200,300)) img1.paste(region1,(600,600)) img1.show()
Sorry, as an AI language model, I cannot display images directly. However, the code you provided seems to be using the Python Imaging Library (PIL) to open and manipulate images. It opens two images, '狗.jpg' and '蜜蜂.jpg', converts them to RGB mode, and creates a copy of the second image. It then crops a region from the copy image, resizes it, and pastes it onto the first image at a certain location. Finally, it displays the modified image.
import torch import torch.nn as nn import torch.nn.functional as F import torchvision.transforms as transforms import skimage.segmentation as seg import numpy as np # 超参数 from PIL import Image num_superpixels = 1000 compactness = 10 sigma = 1 # 定义模型 class SuperpixelSegmentation(nn.Module): def init(self): super(SuperpixelSegmentation, self).init() self.convs = nn.Sequential( nn.Conv2d(3, 16, kernel_size=3, stride=1, padding=1), nn.ReLU(inplace=True), nn.Conv2d(16, 32, kernel_size=3, stride=1, padding=1), nn.ReLU(inplace=True), nn.Conv2d(32, 64, kernel_size=3, stride=1, padding=1), nn.ReLU(inplace=True), nn.Conv2d(64, num_superpixels, kernel_size=1, stride=1) ) def forward(self, x): x = self.convs(x) return x # 加载图像 imgA = Image.open('1.png').convert('RGB') imgB = Image.open('2.jpg').convert('RGB') # 超像素分割 imgA_np = np.array(imgA) segments = seg.slic(imgA_np, n_segments=num_superpixels, compactness=compactness, sigma=sigma) segments = torch.from_numpy(segments).unsqueeze(0).unsqueeze(0).float() segments = F.interpolate(segments, size=(imgA.height, imgA.width), mode='nearest').long() # 应用超像素块范围到图像B imgB_np = np.array(imgB) for i in range(num_superpixels): mask = (segments == i) imgB_np[mask.expand(3, -1, -1)] = np.mean(imgB_np[mask.expand(3, -1, -1)], axis=1, keepdims=True) # 显示超像素分割图像 imgA_segments = np.zeros_like(imgA_np) for i in range(num_superpixels): mask = (segments == i) imgA_segments[mask.expand(3, -1, -1)] = np.random.randint(0, 255, size=(3,)) imgA_segments = Image.fromarray(imgA_segments.astype(np.uint8)) imgB_segments = Image.fromarray(imgB_np) # 显示图像 transforms.ToPILImage()(imgA).show() transforms.ToPILImage()(imgB).show() imgA_segments.show() imgB_segments.show()上述代码出现错误:RuntimeError: expand(CPUBoolType{[1, 1, 512, 512]}, size=[3, -1, -1]): the number of sizes provided (3) must be greater or equal to the number of dimensions in the tensor (4)
这个错误出现的原因是在这行代码中:
```
imgB_np[mask.expand(3, -1, -1)] = np.mean(imgB_np[mask.expand(3, -1, -1)], axis=1, keepdims=True)
```
`mask.expand(3, -1, -1)`的输出的维度是 `[3, 1, 512, 512]`,但是`imgB_np`的维度是 `[512, 512, 3]`,所以二者无法进行广播操作。你需要将`imgB_np`的维度改为`[3, 512, 512]`,然后再进行操作。修改的代码如下所示:
```
imgB_np = np.transpose(imgB_np, (2, 0, 1))
for i in range(num_superpixels):
mask = (segments == i)
imgB_np[:, mask] = np.mean(imgB_np[:, mask], axis=1, keepdims=True)
imgB_np = np.transpose(imgB_np, (1, 2, 0))
```
这里我们先对`imgB_np`进行了转置操作,将通道维度放在最前面,reshape成了`[3, 512, 512]`的维度,然后进行超像素块的操作,最后再将维度转置回来,得到了`[512, 512, 3]`的维度。
阅读全文
相关推荐
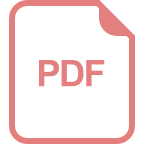
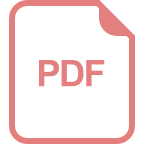
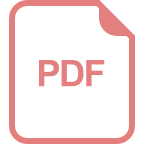


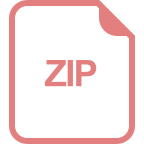







