样本集是jingkou_roi.shp,遥感影像是jingkou9chuli.tif,用python写一段使用最佳指数因子实现特征筛选的代码
时间: 2024-02-09 07:10:20 浏览: 38
好的,以下是一份使用最佳指数因子实现特征筛选的 Python 代码:
```
import gdal
import ogr
import numpy as np
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
from sklearn.model_selection import train_test_split
# 读取遥感影像
img = gdal.Open('jingkou9chuli.tif')
img_width = img.RasterXSize
img_height = img.RasterYSize
img_bands = img.RasterCount
# 读取样本集
shp = ogr.Open('jingkou_roi.shp')
layer = shp.GetLayer()
num_samples = layer.GetFeatureCount()
samples = np.zeros((num_samples, img_bands + 1))
for i in range(num_samples):
feat = layer.GetFeature(i)
for j in range(img_bands):
samples[i][j] = img.ReadAsArray(feat.geometry().GetX(), feat.geometry().GetY(), 1, 1, j, 1)[0][0]
samples[i][img_bands] = feat.GetField('class')
# 划分训练集和测试集
X = samples[:, :-1]
y = samples[:, -1]
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# 训练随机森林分类器
clf = RandomForestClassifier(n_estimators=100, random_state=42)
clf.fit(X_train, y_train)
# 计算特征重要性
importances = clf.feature_importances_
# 根据特征重要性排序
sorted_indices = np.argsort(importances)[::-1]
# 计算最佳指数因子
total_importance = np.sum(importances)
cumulative_importance = 0
best_index_factor = 0
for i in range(img_bands):
cumulative_importance += importances[sorted_indices[i]]
if cumulative_importance / total_importance > (i + 1) / img_bands:
best_index_factor = i + 1
break
# 输出最佳指数因子
print('Best index factor:', best_index_factor)
# 选择最佳指数因子的特征进行分类
X_train_new = X_train[:, sorted_indices[:best_index_factor]]
X_test_new = X_test[:, sorted_indices[:best_index_factor]]
clf_new = RandomForestClassifier(n_estimators=100, random_state=42)
clf_new.fit(X_train_new, y_train)
y_pred = clf_new.predict(X_test_new)
accuracy = accuracy_score(y_test, y_pred)
# 输出分类精度
print('Accuracy:', accuracy)
```
代码中首先读取了遥感影像和样本集,然后使用随机森林分类器计算特征重要性。接着,对特征重要性进行排序,根据最佳指数因子的定义,计算最佳指数因子的值。最后,选择最佳指数因子的特征进行分类,并输出分类精度。
相关推荐




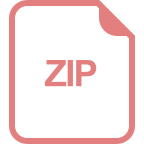
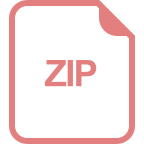
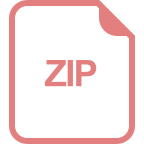
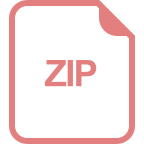
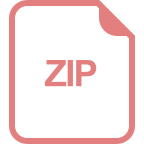
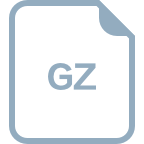
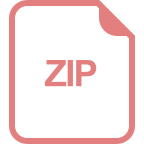
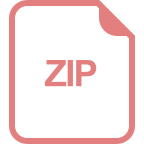
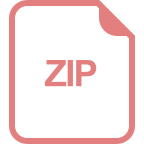
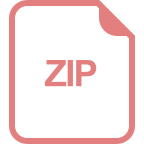