用C语言写一个停车场收费系统
时间: 2023-12-11 18:03:19 浏览: 147
好的,下面是一个基于C语言的简单停车场收费系统的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
struct car {
char licensePlate[10];
time_t enterTime;
time_t exitTime;
int parkingTime;
float fee;
};
void park(struct car *parkingLot, int *parkedCars, int *totalCars);
void leave(struct car *parkingLot, int *parkedCars);
void display(struct car *parkingLot, int *parkedCars);
int main() {
struct car parkingLot[50];
int parkedCars = 0, totalCars = 0, choice;
do {
printf("\n1. Park a vehicle\n");
printf("2. Leave the parking lot\n");
printf("3. Display parking lot status\n");
printf("4. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch(choice) {
case 1:
park(parkingLot, &parkedCars, &totalCars);
break;
case 2:
leave(parkingLot, &parkedCars);
break;
case 3:
display(parkingLot, &parkedCars);
break;
case 4:
printf("\nExiting...");
break;
default:
printf("\nInvalid choice. Please try again.");
}
} while(choice != 4);
return 0;
}
void park(struct car *parkingLot, int *parkedCars, int *totalCars) {
if(*parkedCars < 50) {
printf("\nEnter the license plate number: ");
scanf("%s", parkingLot[*totalCars].licensePlate);
time(&parkingLot[*totalCars].enterTime);
printf("\nVehicle parked successfully.");
*parkedCars += 1;
*totalCars += 1;
} else {
printf("\nSorry, parking lot is full.");
}
}
void leave(struct car *parkingLot, int *parkedCars) {
int i, index = -1;
char licensePlate[10];
time_t currentTime;
float feePerHour = 2.0; // change this to set fee per hour
printf("\nEnter the license plate number: ");
scanf("%s", licensePlate);
time(¤tTime);
for(i = 0; i < *parkedCars; i++) {
if(strcmp(licensePlate, parkingLot[i].licensePlate) == 0) {
index = i;
break;
}
}
if(index != -1) {
parkingLot[index].exitTime = currentTime;
parkingLot[index].parkingTime = (int)difftime(parkingLot[index].exitTime, parkingLot[index].enterTime) / 3600;
parkingLot[index].fee = parkingLot[index].parkingTime * feePerHour;
printf("\nVehicle left the parking lot. Parking fee: %.2f", parkingLot[index].fee);
for(i = index; i < *parkedCars - 1; i++) {
parkingLot[i] = parkingLot[i + 1];
}
*parkedCars -= 1;
} else {
printf("\nVehicle not found in the parking lot.");
}
}
void display(struct car *parkingLot, int *parkedCars) {
int i;
printf("\nTotal parked cars: %d\n", *parkedCars);
printf("License plate number\tEnter time\tParking time\tParking fee\n");
for(i = 0; i < *parkedCars; i++) {
printf("%s\t\t\t%ld\t%d hours\t\t%.2f\n", parkingLot[i].licensePlate, parkingLot[i].enterTime, parkingLot[i].parkingTime, parkingLot[i].fee);
}
}
```
这个程序中,我们定义了一个结构体 `car` 来存储车辆信息,包括车牌号、进入时间、离开时间、停车时间和停车费用。
程序主要分为三个功能模块:停车、离开和显示停车场状态。在停车功能中,我们使用文件操作来记录车辆信息和费用信息。在离开功能中,我们计算并输出车主的停车费用。在显示停车场状态功能中,我们输出当前停车场内所有车辆的信息。
注意:这个示例代码只是一个简单的停车场收费系统,实际应用中可能需要更多的功能和优化,例如多种收费标准、多种支付方式等。
阅读全文
相关推荐






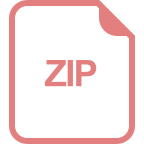
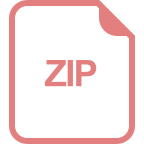
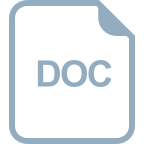





