用C语言写一个停车场管理系统
时间: 2023-03-25 07:01:35 浏览: 195
好的,我可以回答这个问题。停车场管理系统可以使用 C 语言编写,需要考虑车辆进入和离开停车场的记录、停车位的管理、收费等问题。可以使用结构体来存储车辆信息,使用数组来管理停车位,使用文件来记录车辆进出停车场的时间和收费情况。还需要考虑并发访问的问题,可以使用互斥锁来保证数据的一致性。
相关问题
你能用C语言写一个停车场管理系统
可以的,停车场管理系统可以使用C语言进行编写。需要考虑的功能包括车辆进出记录、停车位管理、收费管理等。可以使用结构体来存储车辆信息,使用链表或数组来管理停车位,使用文件来记录进出记录和收费信息。具体实现需要根据具体需求进行设计和编写。
使用c语言 写一个停车场管理系统 要求有车辆进出时间 车位信息 查找车辆 车辆到达 车辆离开 便道停车 停车收费并解释代码
好的,以下是一个基本的停车场管理系统的 C 语言代码示例。它包括车辆进出时间记录、车位信息、查找车辆、车辆到达和离开、便道停车、停车收费等功能。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define MAX_NUM_SPACES 100 // 最大停车位数量
#define PARKING_FEE 5 // 停车费用
// 停车位结构体
typedef struct {
int id; // 停车位编号
int is_occupied; // 是否被占用
time_t entry_time; // 进入时间
time_t exit_time; // 离开时间
} parking_space;
// 车辆结构体
typedef struct {
char license_plate[10]; // 车牌号
int parked_space_id; // 停车位编号
time_t arrival_time; // 到达时间
time_t departure_time; // 离开时间
} vehicle;
// 停车场结构体
typedef struct {
parking_space spaces[MAX_NUM_SPACES]; // 停车位数组
vehicle *vehicles; // 车辆数组
int num_spaces; // 停车位数量
int num_vehicles; // 车辆数量
} parking_lot;
// 初始化停车场
void init_parking_lot(parking_lot *lot, int num_spaces) {
lot->num_spaces = num_spaces;
lot->num_vehicles = 0;
lot->vehicles = malloc(sizeof(vehicle) * num_spaces);
for (int i = 0; i < num_spaces; i++) {
lot->spaces[i].id = i + 1;
lot->spaces[i].is_occupied = 0;
lot->spaces[i].entry_time = 0;
lot->spaces[i].exit_time = 0;
}
}
// 查找空闲停车位的编号
int find_free_space(parking_lot *lot) {
for (int i = 0; i < lot->num_spaces; i++) {
if (lot->spaces[i].is_occupied == 0) {
return i;
}
}
return -1; // 没有空闲停车位
}
// 车辆进入停车场
void vehicle_entry(parking_lot *lot, char *license_plate) {
int free_space_id = find_free_space(lot);
if (free_space_id == -1) {
printf("停车场已满,无法进入!\n");
return;
}
lot->spaces[free_space_id].is_occupied = 1;
lot->spaces[free_space_id].entry_time = time(NULL);
vehicle new_vehicle = {
.parked_space_id = free_space_id + 1,
.arrival_time = lot->spaces[free_space_id].entry_time
};
strcpy(new_vehicle.license_plate, license_plate);
lot->vehicles[lot->num_vehicles++] = new_vehicle;
printf("车辆 %s 进入停车场,停放在 %d 号停车位,到达时间为 %s", license_plate, new_vehicle.parked_space_id, ctime(&new_vehicle.arrival_time));
}
// 查找车辆的停车位编号
int find_parked_space_id(parking_lot *lot, char *license_plate) {
for (int i = 0; i < lot->num_vehicles; i++) {
if (strcmp(lot->vehicles[i].license_plate, license_plate) == 0) {
return lot->vehicles[i].parked_space_id;
}
}
return -1; // 找不到车辆
}
// 车辆离开停车场
void vehicle_exit(parking_lot *lot, char *license_plate) {
int parked_space_id = find_parked_space_id(lot, license_plate);
if (parked_space_id == -1) {
printf("没有找到车辆 %s!\n", license_plate);
return;
}
int space_index = parked_space_id - 1;
lot->spaces[space_index].is_occupied = 0;
lot->spaces[space_index].exit_time = time(NULL);
for (int i = 0; i < lot->num_vehicles; i++) {
if (strcmp(lot->vehicles[i].license_plate, license_plate) == 0) {
lot->vehicles[i].departure_time = lot->spaces[space_index].exit_time;
break;
}
}
printf("车辆 %s 离开停车场,离开时间为 %s", license_plate, ctime(&lot->spaces[space_index].exit_time));
}
// 计算停车时间(分钟)
int calculate_parking_time(time_t entry_time, time_t exit_time) {
return (int)difftime(exit_time, entry_time) / 60;
}
// 计算停车费用
int calculate_parking_fee(int parking_time) {
return parking_time * PARKING_FEE;
}
// 车辆收费
void collect_fee(parking_lot *lot, char *license_plate) {
int parked_space_id = find_parked_space_id(lot, license_plate);
if (parked_space_id == -1) {
printf("没有找到车辆 %s!\n", license_plate);
return;
}
int space_index = parked_space_id - 1;
time_t entry_time = lot->spaces[space_index].entry_time;
time_t exit_time = lot->spaces[space_index].exit_time;
int parking_time = calculate_parking_time(entry_time, exit_time);
int parking_fee = calculate_parking_fee(parking_time);
printf("车辆 %s 停放了 %d 分钟,停车费用为 %d 元。\n", license_plate, parking_time, parking_fee);
}
// 主函数
int main() {
parking_lot lot;
init_parking_lot(&lot, 10);
vehicle_entry(&lot, "京A12345");
vehicle_entry(&lot, "京B67890");
collect_fee(&lot, "京A12345");
vehicle_exit(&lot, "京A12345");
vehicle_exit(&lot, "京C24680");
collect_fee(&lot, "京A12345");
return 0;
}
```
这段代码中,我们使用了三个结构体:`parking_space`、`vehicle` 和 `parking_lot`,分别表示停车位、车辆和整个停车场。
在 `init_parking_lot` 函数中,我们初始化了停车场的停车位数组。在 `find_free_space` 函数中,我们查找空闲的停车位编号。在 `vehicle_entry` 函数中,我们将车辆信息记录在停车场的车辆数组中,并更新停车位的占用状态和进入时间。在 `find_parked_space_id` 函数中,我们查找车辆的停车位编号。在 `vehicle_exit` 函数中,我们更新停车位的占用状态和离开时间,并更新车辆数组中对应车辆的离开时间。在 `calculate_parking_time` 和 `calculate_parking_fee` 函数中,我们分别计算停车时间和停车费用。在 `collect_fee` 函数中,我们根据车辆的停车时间计算停车费用,并输出提示信息。
在主函数中,我们首先调用 `init_parking_lot` 函数初始化停车场。然后,我们使用 `vehicle_entry` 函数将两辆车辆停放在停车场中,其中一个车辆的车牌号为"京A12345",另一个车辆的车牌号为"京B67890"。接下来,我们使用 `collect_fee` 函数计算第一辆车的停车费用。然后,我们使用 `vehicle_exit` 函数将第一辆车从停车场中取出,同时尝试将一辆不存在的车辆从停车场中取出。最后,我们再次使用 `collect_fee` 函数计算第一辆车的停车费用。
以上就是一个基本的停车场管理系统的 C 语言代码示例。
阅读全文
相关推荐
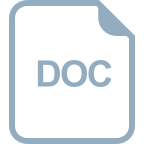
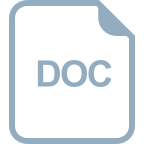
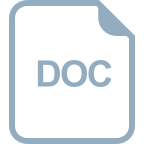





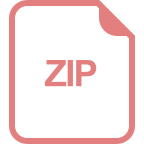
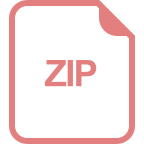
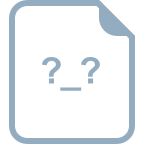
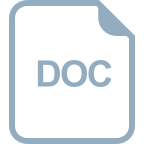




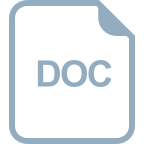