请用C语言写出停车场管理系统
时间: 2024-10-12 11:01:09 浏览: 68
创建一个简单的停车场管理系统涉及到文件操作、数据结构和用户交互等功能,下面是一个简化的示例,使用C语言的基本控制结构和数组来表示停车位:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_PARKINGSLOTS 10 // 停车位最大数量
typedef struct {
int slot_number;
char is_empty; // 0 表示空位,1 表示已占用
} ParkingSlot;
// 函数声明
ParkingSlot* create_slot();
int park_car(int slot);
void display_status();
int main() {
ParkingSlot parkinglots[MAX_PARKINGSLOTS] = { {0, 1} }; // 初始化第一个停车位为已占用
int choice;
while (1) {
printf("停车场状态:\n");
for (int i = 0; i < MAX_PARKINGSLOTS; i++) {
if (parkinglots[i].is_empty)
printf("%d. 空\n", i + 1);
else
printf("%d. 已停车\n", i + 1);
}
printf("\n选择操作(1: 进场,2: 查看,3: 退出):\n");
scanf("%d", &choice);
switch (choice) {
case 1:
park_car(parkinglots);
break;
case 2:
display_status();
break;
case 3:
exit(0); // 退出程序
default:
printf("无效选择,请重新输入。\n");
}
}
return 0;
}
// 创建停车位函数
ParkingSlot* create_slot() {
for (int i = 0; i < MAX_PARKINGSLOTS; i++) {
if (parkinglots[i].is_empty) {
parkinglots[i].slot_number = i + 1;
parkinglots[i].is_empty = 0;
return &parkinglots[i];
}
}
return NULL; // 没有空位了
}
// 将车停入停车位
int park_car(int slot_num) {
if (slot_num > 0 && slot_num <= MAX_PARKINGSLOTS && parkinglots[slot_num - 1].is_empty) {
parkinglots[slot_num - 1].is_empty = 0;
printf("车已停放至停车位 %d.\n", slot_num);
return 1;
} else {
printf("该停车位不可用。\n");
return 0;
}
}
// 显示当前停车位状态
void display_status() {
for (int i = 0; i < MAX_PARKINGSLOTS; i++) {
printf("停车位 %d: %s\n", parkinglots[i].slot_number, parkinglots[i].is_empty ? "空" : "已停车");
}
}
```
这个简单的示例仅作为起点,实际应用中可能需要更复杂的数据结构、数据库支持以及错误处理。请注意,这个代码并没有考虑并发访问的问题。
阅读全文
相关推荐
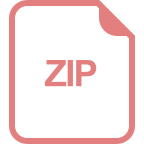
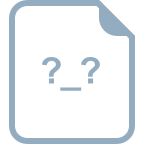
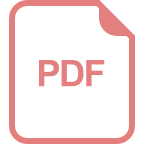
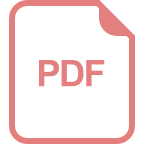
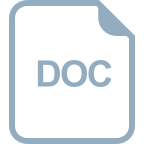
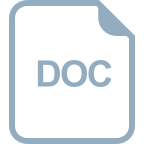
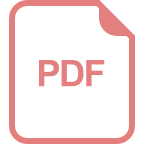
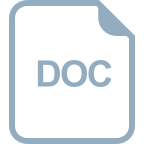
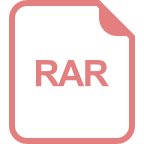
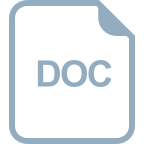
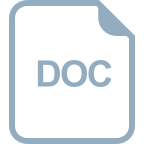
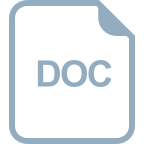
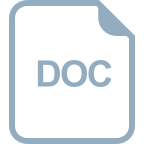
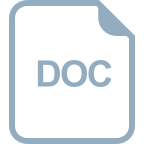




