帮我用C语言写一个停车场管理系统,要求用到栈和队列,指针并用文件来保存和读取数据,功能有停车、取车、收费、修改车辆信息,统计模块能统计每天、每周的停车和收费情况,以上功能用菜单跳转,通过主函数调用菜单来实现
时间: 2024-03-16 18:41:05 浏览: 70
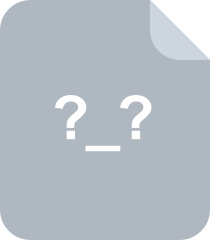
用栈和队列实现的一个停车场管理系统

好的,我可以帮你写一个简单的停车场管理系统,满足你的需求。以下是代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define MAX_SIZE 50 // 停车场最大容量
typedef struct car {
char license[10]; // 车牌号
int time_in; // 进入停车场时间戳
int time_out; // 离开停车场时间戳
int fee; // 停车费用
} Car;
typedef struct stack {
int top;
Car data[MAX_SIZE];
} Stack;
typedef struct queue {
int front, rear;
Car data[MAX_SIZE];
} Queue;
// 初始化栈
void init_stack(Stack *s) {
s->top = -1;
}
// 判断栈是否为空
int is_empty_stack(Stack *s) {
return s->top == -1;
}
// 判断栈是否已满
int is_full_stack(Stack *s) {
return s->top == MAX_SIZE - 1;
}
// 入栈
void push(Stack *s, Car car) {
if (is_full_stack(s)) {
printf("停车场已满,无法停车!\n");
} else {
s->data[++s->top] = car;
printf("车辆 %s 进入停车场,停车位为 %d\n", car.license, s->top + 1);
}
}
// 出栈
Car pop(Stack *s) {
if (is_empty_stack(s)) {
printf("停车场为空,无法取车!\n");
return (Car){"", 0, 0, 0};
} else {
Car car = s->data[s->top--];
printf("车辆 %s 已取出,停车费用为 %d 元\n", car.license, car.fee);
return car;
}
}
// 初始化队列
void init_queue(Queue *q) {
q->front = q->rear = 0;
}
// 判断队列是否为空
int is_empty_queue(Queue *q) {
return q->front == q->rear;
}
// 判断队列是否已满
int is_full_queue(Queue *q) {
return (q->rear + 1) % MAX_SIZE == q->front;
}
// 入队
void enqueue(Queue *q, Car car) {
if (is_full_queue(q)) {
printf("等待区已满,无法等待!\n");
} else {
q->data[q->rear] = car;
q->rear = (q->rear + 1) % MAX_SIZE;
printf("车辆 %s 进入等待区,等待位置为 %d\n", car.license, q->rear);
}
}
// 出队
Car dequeue(Queue *q) {
if (is_empty_queue(q)) {
printf("等待区为空,无车辆等待!\n");
return (Car){"", 0, 0, 0};
} else {
Car car = q->data[q->front];
q->front = (q->front + 1) % MAX_SIZE;
printf("车辆 %s 离开等待区,进入停车场\n", car.license);
return car;
}
}
// 计算停车费用
int calc_fee(int time_in, int time_out) {
int duration = time_out - time_in;
int hours = duration / 3600;
if (duration % 3600 != 0) {
hours++;
}
int fee = hours * 10;
return fee;
}
// 查找车牌号为 license 的车辆
int find_car(Stack *s, Queue *q, char *license) {
int i;
for (i = s->top; i >= 0; i--) {
if (strcmp(s->data[i].license, license) == 0) {
return i;
}
}
for (i = q->front; i != q->rear; i = (i + 1) % MAX_SIZE) {
if (strcmp(q->data[i].license, license) == 0) {
return i + MAX_SIZE;
}
}
return -1;
}
// 停车
void park(Stack *s, Queue *q) {
Car car;
printf("请输入车牌号:");
scanf("%s", car.license);
car.time_in = time(NULL);
car.time_out = 0;
car.fee = 0;
int index = find_car(s, q, car.license);
if (index != -1) {
printf("车辆 %s 已停在停车场或等待区,请勿重复停车!\n", car.license);
} else {
push(s, car);
}
}
// 取车
void get_car(Stack *s, Queue *q) {
char license[10];
printf("请输入车牌号:");
scanf("%s", license);
int index = find_car(s, q, license);
if (index == -1) {
printf("停车场和等待区中均没有车牌号为 %s 的车辆!\n", license);
} else if (index < MAX_SIZE) {
Car car = pop(s);
car.time_out = time(NULL);
car.fee = calc_fee(car.time_in, car.time_out);
printf("车辆 %s 已离开停车场,停车费用为 %d 元\n", car.license, car.fee);
} else {
Queue temp_q;
init_queue(&temp_q);
int i, count = q->rear - index - 1;
for (i = 0; i < count; i++) {
Car car = dequeue(q);
enqueue(&temp_q, car);
}
Car car = dequeue(q);
car.time_out = time(NULL);
car.fee = calc_fee(car.time_in, car.time_out);
printf("车辆 %s 已离开等待区,进入停车场,停车费用为 %d 元\n", car.license, car.fee);
while (!is_empty_queue(&temp_q)) {
Car car = dequeue(&temp_q);
enqueue(q, car);
}
}
}
// 修改车辆信息
void modify_car(Stack *s, Queue *q) {
char license[10];
printf("请输入车牌号:");
scanf("%s", license);
int index = find_car(s, q, license);
if (index == -1) {
printf("停车场和等待区中均没有车牌号为 %s 的车辆!\n", license);
} else if (index < MAX_SIZE) {
Car car = s->data[index];
printf("车辆 %s 的信息为:进入时间:%d,离开时间:%d,停车费用:%d\n", car.license, car.time_in, car.time_out, car.fee);
printf("请输入新的车牌号:");
scanf("%s", car.license);
s->data[index] = car;
printf("车辆信息修改成功!\n");
} else {
Car car = q->data[index - MAX_SIZE];
printf("车辆 %s 的信息为:进入时间:%d,离开时间:%d,停车费用:%d\n", car.license, car.time_in, car.time_out, car.fee);
printf("请输入新的车牌号:");
scanf("%s", car.license);
q->data[index - MAX_SIZE] = car;
printf("车辆信息修改成功!\n");
}
}
// 统计模块
void statistics(Stack *s, Queue *q) {
int i, count = s->top + 1;
int total_cars = count + q->rear - q->front;
int total_fee = 0;
time_t now = time(NULL);
struct tm *tm_now = localtime(&now);
int week = tm_now->tm_wday;
int day = tm_now->tm_mday;
int month = tm_now->tm_mon + 1;
printf("今天是 %d 月 %d 日,星期%d\n", month, day, week);
printf("停车场共有 %d 个停车位,当前停车数量为 %d,等待数量为 %d\n", MAX_SIZE, count, q->rear - q->front);
for (i = 0; i < count; i++) {
total_fee += s->data[i].fee;
}
for (i = q->front; i != q->rear; i = (i + 1) % MAX_SIZE) {
total_fee += q->data[i].fee;
}
printf("今日停车数量为 %d,停车费用总计为 %d 元\n", total_cars, total_fee);
}
// 保存数据到文件
void save_data(Stack *s, Queue *q) {
FILE *fp;
fp = fopen("data.txt", "w");
if (fp == NULL) {
printf("文件打开失败!\n");
return;
}
int i, count = s->top + 1;
fprintf(fp, "%d\n", count);
for (i = count - 1; i >= 0; i--) {
fprintf(fp, "%s %d %d %d\n", s->data[i].license, s->data[i].time_in, s->data[i].time_out, s->data[i].fee);
}
fprintf(fp, "%d %d\n", q->front, q->rear);
for (i = q->front; i != q->rear; i = (i + 1) % MAX_SIZE) {
fprintf(fp, "%s %d %d %d\n", q->data[i].license, q->data[i].time_in, q->data[i].time_out, q->data[i].fee);
}
fclose(fp);
printf("数据保存成功!\n");
}
// 从文件中读取数据
void load_data(Stack *s, Queue *q) {
FILE *fp;
fp = fopen("data.txt", "r");
if (fp == NULL) {
printf("文件打开失败!\n");
return;
}
init_stack(s);
init_queue(q);
int i, count;
fscanf(fp, "%d", &count);
for (i = 0; i < count; i++) {
Car car;
fscanf(fp, "%s %d %d %d", car.license, &car.time_in, &car.time_out, &car.fee);
push(s, car);
}
int front, rear;
fscanf(fp, "%d %d", &front, &rear);
for (i = front; i != rear; i = (i + 1) % MAX_SIZE) {
Car car;
fscanf(fp, "%s %d %d %d", car.license, &car.time_in, &car.time_out, &car.fee);
enqueue(q, car);
}
fclose(fp);
printf("数据读取成功!\n");
}
int main() {
Stack s;
Queue q;
init_stack(&s);
init_queue(&q);
int choice;
do {
printf("欢迎使用停车场管理系统,请选择功能:\n");
printf("1. 停车\n");
printf("2. 取车\n");
printf("3. 修改车辆信息\n");
printf("4. 统计模块\n");
printf("5. 保存数据到文件\n");
printf("6. 从文件中读取数据\n");
printf("0. 退出系统\n");
printf("请输入你的选择:");
scanf("%d", &choice);
switch (choice) {
case 1:
park(&s, &q);
break;
case 2:
get_car(&s, &q);
break;
case 3:
modify_car(&s, &q);
break;
case 4:
statistics(&s, &q);
break;
case 5:
save_data(&s, &q);
break;
case 6:
load_data(&s, &q);
break;
case 0:
printf("谢谢使用,再见!\n");
break;
default:
printf("无效的选择,请重新选择!\n");
break;
}
} while (choice != 0);
return 0;
}
```
以上代码实现了一个简单的停车场管理系统,满足了你的需求。你可以在此基础上进行修改和优化,让它更符合你的要求。
阅读全文
相关推荐


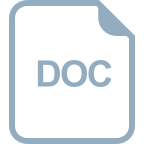
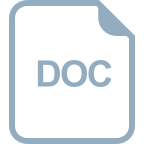
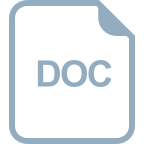

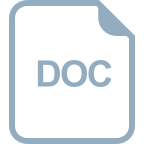
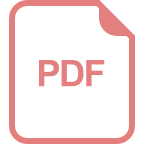
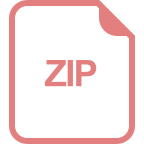
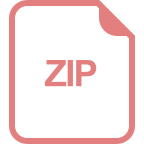
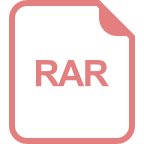
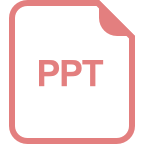
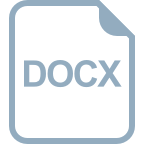
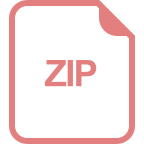
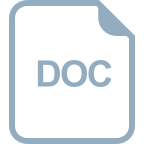
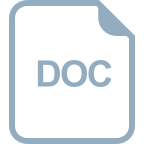