编写函数,生成 20 次随机掷骰子的序列,将它们存储在一个列表中,然后输出骰子值, # 输出时要求将连续出现次数最多的骰子值包含在括号中,如下所示: # 1 2 5 5 3 1 2 4 3 (2 2 2 2) 3 6 5 5 6 3 1
时间: 2024-01-07 16:02:56 浏览: 55
可以使用Python的`random`模块来模拟掷骰子的过程。下面是实现的代码:
```python
import random
def roll_dice():
dice_sequence = []
for i in range(20):
dice_sequence.append(random.randint(1, 6))
max_count = 0
current_count = 1
max_value = 0
for i in range(1, len(dice_sequence)):
if dice_sequence[i] == dice_sequence[i-1]:
current_count += 1
if current_count > max_count:
max_count = current_count
max_value = dice_sequence[i]
else:
current_count = 1
output_sequence = []
for i in range(len(dice_sequence)):
if i == max_count-1:
output_sequence.append('(' + str(dice_sequence[i]))
elif i == max_count:
output_sequence.append(str(dice_sequence[i]) + ')')
else:
output_sequence.append(str(dice_sequence[i]))
output_string = ' '.join(output_sequence)
return output_string
```
这个函数会生成20次随机掷骰子的序列,并将它们存储在一个列表中。然后,它会找出连续出现次数最多的骰子值,并将其包含在括号中。最后,它将输出一个由空格分隔的字符串,表示骰子的序列。
使用示例:
```python
dice_sequence = roll_dice()
print(dice_sequence)
```
输出结果类似于:
```
5 3 1 6 5 2 4 5 5 (4 4 4) 1 3 2 2 3 2 6 4 4 3
```
其中,括号中的数字表示连续出现次数最多的骰子值。
阅读全文
相关推荐


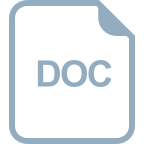
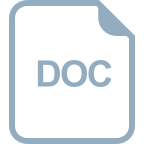
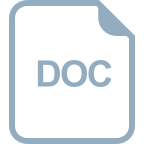
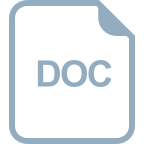
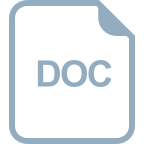
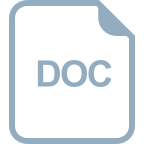
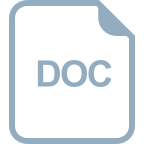
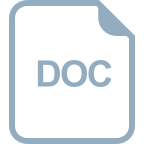
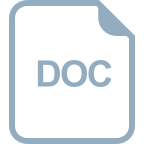
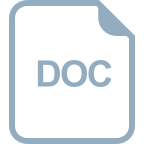
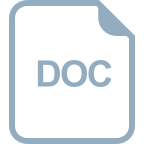
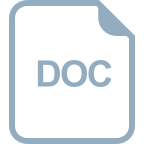
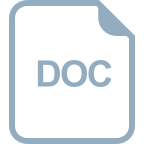
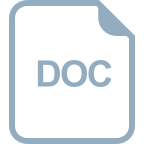