用C语言写一个图书信息管理系统设计
时间: 2023-12-29 10:16:51 浏览: 84
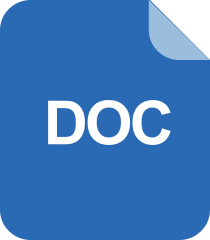
《C语言程序设计》图书信息管理系统.doc
好的,下面是一个简单的用C语言编写的图书信息管理系统设计:
1. 定义图书信息结构体
```c
typedef struct Book {
int id; // 图书ID
char title[50]; // 图书名称
char author[30]; // 作者
char publisher[30]; // 出版社
int year; // 出版年份
int quantity; // 库存量
} Book;
```
2. 实现基本操作函数
```c
// 添加图书信息
void add_book(Book *books, int *count) {
printf("Please enter the book ID: ");
scanf("%d", &books[*count].id);
printf("Please enter the book title: ");
scanf("%s", books[*count].title);
printf("Please enter the author: ");
scanf("%s", books[*count].author);
printf("Please enter the publisher: ");
scanf("%s", books[*count].publisher);
printf("Please enter the publication year: ");
scanf("%d", &books[*count].year);
printf("Please enter the quantity: ");
scanf("%d", &books[*count].quantity);
(*count)++;
}
// 删除指定ID的图书信息
void delete_book(Book *books, int *count, int id) {
for (int i = 0; i < *count; i++) {
if (books[i].id == id) {
for (int j = i; j < *count - 1; j++) {
books[j] = books[j + 1];
}
(*count)--;
printf("Book with ID %d has been deleted.\n", id);
return;
}
}
printf("Book with ID %d is not found.\n", id);
}
// 显示所有图书信息
void display_books(Book *books, int count) {
printf("%-10s %-50s %-30s %-30s %-10s %-10s\n", "ID", "Title", "Author", "Publisher", "Year", "Quantity");
for (int i = 0; i < count; i++) {
printf("%-10d %-50s %-30s %-30s %-10d %-10d\n", books[i].id, books[i].title, books[i].author, books[i].publisher, books[i].year, books[i].quantity);
}
}
// 按照图书ID或名称搜索图书信息
void search_book(Book *books, int count) {
int choice, id;
char title[50];
printf("1. Search by ID\n");
printf("2. Search by title\n");
printf("Please enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("Please enter the book ID: ");
scanf("%d", &id);
for (int i = 0; i < count; i++) {
if (books[i].id == id) {
printf("%-10s %-50s %-30s %-30s %-10s %-10s\n", "ID", "Title", "Author", "Publisher", "Year", "Quantity");
printf("%-10d %-50s %-30s %-30s %-10d %-10d\n", books[i].id, books[i].title, books[i].author, books[i].publisher, books[i].year, books[i].quantity);
return;
}
}
printf("Book with ID %d is not found.\n", id);
break;
case 2:
printf("Please enter the book title: ");
scanf("%s", title);
for (int i = 0; i < count; i++) {
if (strcmp(books[i].title, title) == 0) {
printf("%-10s %-50s %-30s %-30s %-10s %-10s\n", "ID", "Title", "Author", "Publisher", "Year", "Quantity");
printf("%-10d %-50s %-30s %-30s %-10d %-10d\n", books[i].id, books[i].title, books[i].author, books[i].publisher, books[i].year, books[i].quantity);
return;
}
}
printf("Book with title %s is not found.\n", title);
break;
default:
printf("Invalid choice.\n");
break;
}
}
// 借阅指定ID的图书
void borrow_book(Book *books, int count, int id) {
for (int i = 0; i < count; i++) {
if (books[i].id == id) {
if (books[i].quantity > 0) {
books[i].quantity--;
printf("Book with ID %d has been borrowed.\n", id);
} else {
printf("Book with ID %d is out of stock.\n", id);
}
return;
}
}
printf("Book with ID %d is not found.\n", id);
}
// 归还指定ID的图书
void return_book(Book *books, int count, int id) {
for (int i = 0; i < count; i++) {
if (books[i].id == id) {
books[i].quantity++;
printf("Book with ID %d has been returned.\n", id);
return;
}
}
printf("Book with ID %d is not found.\n", id);
}
```
3. 实现文件读写功能
```c
// 将图书信息写入文件
void write_books_to_file(Book *books, int count, char *filename) {
FILE *fp = fopen(filename, "w");
if (fp == NULL) {
printf("Failed to open file %s\n", filename);
return;
}
fprintf(fp, "%d\n", count);
for (int i = 0; i < count; i++) {
fprintf(fp, "%d %s %s %s %d %d\n", books[i].id, books[i].title, books[i].author, books[i].publisher, books[i].year, books[i].quantity);
}
fclose(fp);
}
// 从文件中读取图书信息
int read_books_from_file(Book *books, char *filename) {
FILE *fp = fopen(filename, "r");
if (fp == NULL) {
printf("Failed to open file %s\n", filename);
return 0;
}
int count;
fscanf(fp, "%d\n", &count);
for (int i = 0; i < count; i++) {
fscanf(fp, "%d %s %s %s %d %d\n", &books[i].id, books[i].title, books[i].author, books[i].publisher, &books[i].year, &books[i].quantity);
}
fclose(fp);
return count;
}
```
4. 实现主函数
```c
int main() {
Book books[100];
int count = 0;
count = read_books_from_file(books, "books.txt");
int choice, id;
while (1) {
choice = main_menu();
switch (choice) {
case 1:
add_book(books, &count);
break;
case 2:
printf("Please enter the book ID: ");
scanf("%d", &id);
delete_book(books, &count, id);
break;
case 3:
display_books(books, count);
break;
case 4:
search_book(books, count);
break;
case 5:
printf("Please enter the book ID: ");
scanf("%d", &id);
borrow_book(books, count, id);
break;
case 6:
printf("Please enter the book ID: ");
scanf("%d", &id);
return_book(books, count, id);
break;
case 7:
write_books_to_file(books, count, "books.txt");
return 0;
default:
printf("Invalid choice.\n");
break;
}
}
return 0;
}
```
希望这个简单的设计可以帮助您实现一个基本的图书信息管理系统。
阅读全文
相关推荐
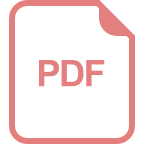




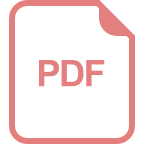
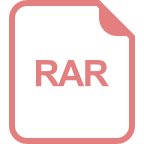
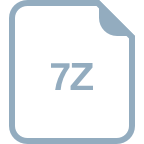
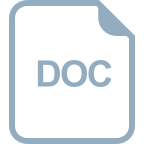


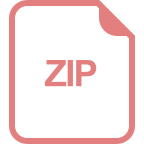
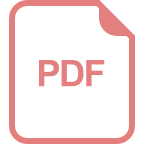