用C语言链表写一个图书馆信息管理系统
时间: 2023-07-18 12:25:23 浏览: 49
好的,下面是一个使用链表实现的图书馆信息管理系统的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_TITLE_LEN 50 // 书名最大长度
#define MAX_AUTHOR_LEN 30 // 作者名最大长度
typedef struct book {
char title[MAX_TITLE_LEN];
char author[MAX_AUTHOR_LEN];
int year;
struct book *next;
} book_t;
book_t *head = NULL; // 链表头指针
void add_book() {
book_t *new_book = (book_t*) malloc(sizeof(book_t));
printf("Enter book title: ");
scanf("%s", new_book->title);
printf("Enter author name: ");
scanf("%s", new_book->author);
printf("Enter year published: ");
scanf("%d", &new_book->year);
new_book->next = NULL;
if (head == NULL) {
head = new_book;
} else {
book_t *cur = head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = new_book;
}
printf("Book added successfully.\n");
}
void search_book() {
char query[MAX_TITLE_LEN];
printf("Enter book title to search: ");
scanf("%s", query);
book_t *cur = head;
int found = 0;
while (cur != NULL) {
if (strcmp(cur->title, query) == 0) {
printf("Book found:\n");
printf("Title: %s\n", cur->title);
printf("Author: %s\n", cur->author);
printf("Year: %d\n", cur->year);
found = 1;
break;
}
cur = cur->next;
}
if (!found) {
printf("Book not found.\n");
}
}
void print_books() {
printf("All books in library:\n");
book_t *cur = head;
int i = 1;
while (cur != NULL) {
printf("%d. %s by %s (%d)\n", i, cur->title, cur->author, cur->year);
i++;
cur = cur->next;
}
}
void free_books() {
book_t *cur = head;
while (cur != NULL) {
book_t *temp = cur;
cur = cur->next;
free(temp);
}
}
int main() {
int choice = 0;
while (choice != 4) {
printf("Library Information Management System\n");
printf("1. Add book\n");
printf("2. Search book\n");
printf("3. Print all books\n");
printf("4. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_book();
break;
case 2:
search_book();
break;
case 3:
print_books();
break;
case 4:
printf("Exiting...\n");
break;
default:
printf("Invalid choice, please try again.\n");
break;
}
}
free_books();
return 0;
}
```
这个示例实现了一个使用链表存储图书信息的图书馆信息管理系统,其中包括添加书籍、搜索书籍和打印所有书籍等功能。你可以根据自己的需求对其进行修改和扩展。需要注意的是,使用链表存储数据时需要注意内存管理,确保在程序结束时释放内存。
相关推荐
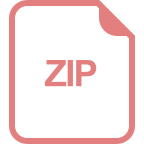
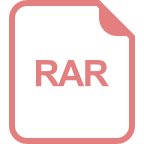
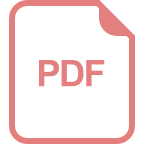














