不使用链表,用C语言编写一个家庭图书管理系统
时间: 2024-03-24 21:40:08 浏览: 70
好的,下面是一个简单的家庭图书管理系统的实现,不使用链表这种数据结构:
```c
#include <stdio.h>
#include <string.h>
#define MAX_BOOKS 100
struct Book {
char title[50];
char author[50];
int year;
int pages;
};
struct Library {
struct Book books[MAX_BOOKS];
int num_books;
};
void init_library(struct Library *lib) {
lib->num_books = 0;
}
void add_book(struct Library *lib, const char *title, const char *author, int year, int pages) {
if (lib->num_books >= MAX_BOOKS) {
printf("The library is full!\n");
return;
}
struct Book *book = &lib->books[lib->num_books++];
strncpy(book->title, title, 49);
strncpy(book->author, author, 49);
book->year = year;
book->pages = pages;
}
void show_books(const struct Library *lib) {
printf("The library has %d books:\n", lib->num_books);
for (int i = 0; i < lib->num_books; i++) {
struct Book book = lib->books[i];
printf("%s by %s, %d pages, published in %d\n", book.title, book.author, book.pages, book.year);
}
}
void search_by_author(const struct Library *lib, const char *author) {
printf("Books by %s:\n", author);
for (int i = 0; i < lib->num_books; i++) {
struct Book book = lib->books[i];
if (strcmp(book.author, author) == 0) {
printf("%s, %d pages, published in %d\n", book.title, book.pages, book.year);
}
}
}
void search_by_title(const struct Library *lib, const char *title) {
printf("Books titled %s:\n", title);
for (int i = 0; i < lib->num_books; i++) {
struct Book book = lib->books[i];
if (strcmp(book.title, title) == 0) {
printf("%s by %s, %d pages, published in %d\n", book.title, book.author, book.pages, book.year);
}
}
}
int main() {
struct Library lib;
init_library(&lib);
add_book(&lib, "The Catcher in the Rye", "J.D. Salinger", 1951, 277);
add_book(&lib, "To Kill a Mockingbird", "Harper Lee", 1960, 281);
add_book(&lib, "1984", "George Orwell", 1949, 328);
show_books(&lib);
search_by_author(&lib, "Harper Lee");
search_by_title(&lib, "1984");
return 0;
}
```
在这个实现中,我们使用了两个结构体,一个是 `Book`,表示一本书,另一个是 `Library`,表示整个图书馆。`Library` 结构体包含一个 `Book` 数组和一个整数 `num_books`,表示图书馆中有多少本书。在 `init_library` 函数中,我们将 `num_books` 初始化为 0。
在 `add_book` 函数中,我们首先检查图书馆是否已满,如果是则打印出一条错误信息,否则将新书添加到 `books` 数组中。注意,我们使用了指针来操作 `books` 数组中的元素,并使用 `strncpy` 函数来复制字符串。
在 `show_books` 函数中,我们遍历 `books` 数组中的每一本书,并打印出书的信息。
在 `search_by_author` 和 `search_by_title` 函数中,我们分别遍历 `books` 数组中的每一本书,如果找到了匹配的作者或书名,则打印出书的信息。
最后,在 `main` 函数中,我们初始化了一个 `Library` 结构体,并添加了三本书。然后分别调用 `show_books`、`search_by_author` 和 `search_by_title` 函数来测试我们的程序。
需要注意的是,这个实现非常简单,没有考虑到很多实际情况,例如如何删除书籍、如何检查输入是否合法、如何保存和读取数据等等。如果您需要一个更完整和健壮的家庭图书管理系统,建议使用链表等数据结构来实现。
阅读全文
相关推荐
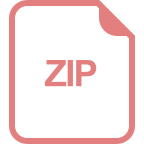
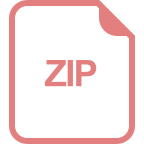
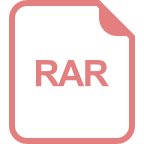















