c语言编写一个用链表实现的图书管理系统的程序
时间: 2024-10-07 10:03:20 浏览: 59
在C语言中,我们可以使用链表数据结构来实现一个简单的图书管理系统。这里提供一个基本的图书节点定义和操作书籍的示例,包括添加书、删除书和查找书的功能。我们将创建一个BookNode结构体作为链表节点,并维护一个BookList类型的链表。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义图书节点结构
typedef struct BookNode {
char title[50];
char author[50];
int id;
struct BookNode* next;
} BookNode;
// 创建新的图书节点
BookNode* create_book_node(char* title, char* author, int id) {
BookNode* new_node = (BookNode*)malloc(sizeof(BookNode));
if (!new_node) {
printf("Memory allocation failed.\n");
return NULL;
}
strcpy(new_node->title, title);
strcpy(new_node->author, author);
new_node->id = id;
new_node->next = NULL;
return new_node;
}
// 添加图书到链表
void add_book(BookList* list, char* title, char* author, int id) {
BookNode* new_book = create_book_node(title, author, id);
if (new_book) {
if (*list == NULL) {
*list = new_book;
} else {
BookNode* current = list;
while (current->next != NULL) {
current = current->next;
}
current->next = new_book;
}
}
}
// 删除图书
void delete_book(BookList* list, int id) {
if (*list == NULL) {
return;
}
BookNode* current = *list;
if (current->id == id) {
*list = current->next;
free(current);
return;
}
while (current->next != NULL && current->next->id != id) {
current = current->next;
}
if (current->next != NULL) {
BookNode* temp = current->next;
current->next = temp->next;
free(temp);
}
}
// 查找图书
BookNode* find_book(BookList* list, int id) {
BookNode* current = *list;
while (current != NULL) {
if (current->id == id) {
return current;
}
current = current->next;
}
return NULL;
}
int main() {
// 初始化链表
BookList book_list = {NULL};
// 添加书籍示例
add_book(&book_list, "Title1", "Author1", 1);
add_book(&book_list, "Title2", "Author2", 2);
// 查找书籍并打印
BookNode* found_book = find_book(&book_list, 1);
if (found_book) {
printf("Found book with id %d: Title - %s, Author - %s\n", found_book->id, found_book->title, found_book->author);
} else {
printf("Book not found.\n");
}
// 删除书籍示例
delete_book(&book_list, 2);
return 0;
}
```
在这个示例中,我们首先定义了链表头指针`BookList`,然后实现了几个基本操作函数。你可以根据实际需求扩展这个基础框架,比如添加搜索所有图书的功能等。
阅读全文
相关推荐
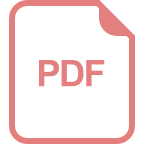
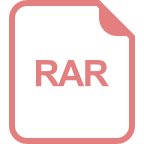



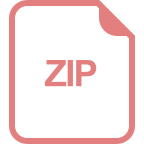
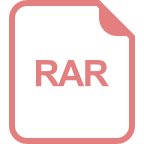
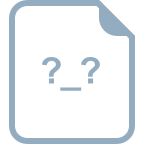
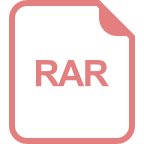
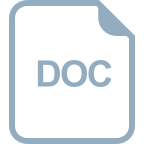
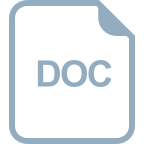
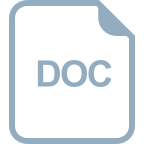
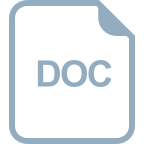
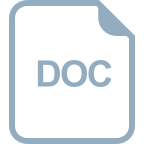
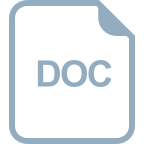
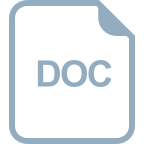
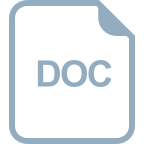
