用python写一个K-means的代码
时间: 2023-09-12 16:05:40 浏览: 58
### 回答1:
好的,下面是一个简单的 Python K-means 实现代码:
```python
import numpy as np
class KMeans:
def __init__(self, n_clusters=8, max_iter=300):
self.n_clusters = n_clusters
self.max_iter = max_iter
def fit(self, X):
n_samples, n_features = X.shape
# 初始化聚类中心
rng = np.random.RandomState(42)
i = rng.permutation(n_samples)[:self.n_clusters]
centers = X[i]
# 迭代优化聚类中心
for _ in range(self.max_iter):
# 计算每个样本到聚类中心的距离
distances = np.sqrt(((X - centers[:, np.newaxis])**2).sum(axis=2))
# 分配样本到最近的聚类中心
labels = np.argmin(distances, axis=0)
# 更新聚类中心
for i in range(self.n_clusters):
centers[i] = X[labels == i].mean(axis=0)
self.labels_ = labels
self.cluster_centers_ = centers
```
其中,`n_clusters` 是指定聚类的个数;`max_iter` 是指定最大迭代次数。`fit` 方法接收一个 $n \times m$ 的数据矩阵 `X`,其中 $n$ 是样本数,$m$ 是特征数。它会返回每个样本所属的聚类 `labels_`,以及每个聚类的中心 `cluster_centers_`。
### 回答2:
K-means是一种常用的聚类算法,它将样本数据分为K个不同的簇,使得簇内的数据点相似度尽可能高,并且不同簇之间的相似度尽可能低。
下面是一个用Python编写的简单K-means算法的代码示例:
```python
import numpy as np
def kmeans(data, K, max_iters):
# 随机选择K个中心点
centers_idx = np.random.choice(len(data), K, replace=False)
centers = data[centers_idx]
for _ in range(max_iters):
# 分配数据点到最近的中心点
clusters = [[] for _ in range(K)]
for point in data:
distances = np.linalg.norm(centers - point, axis=1)
cluster_idx = np.argmin(distances)
clusters[cluster_idx].append(point)
# 更新中心点
for i, cluster in enumerate(clusters):
if len(cluster) > 0:
centers[i] = np.mean(cluster, axis=0)
# 返回簇的标签和中心点
labels = np.zeros(len(data))
for i, cluster in enumerate(clusters):
for point in cluster:
labels[np.where(data == point)[0][0]] = i
return labels, centers
```
这个代码中,首先会随机选择K个中心点,然后迭代K-means算法进行聚类,直到达到最大迭代次数。在每次迭代中,会将数据点分配到最近的中心点,并更新中心点位置。最后返回样本数据的簇标签和中心点位置。
使用这个代码,可以对给定的数据集进行聚类,例如:
```python
from sklearn.datasets import make_blobs
# 生成一个示例数据集
data, _ = make_blobs(n_samples=100, centers=3, random_state=0)
# 调用K-means算法
labels, centers = kmeans(data, K=3, max_iters=10)
print(labels)
print(centers)
```
以上就是一个用Python实现K-means聚类算法的代码示例,其中使用了numpy库进行矩阵运算和数据计算,通过随机选择初始中心点和多次迭代的方式实现了聚类过程。
### 回答3:
K-means是一种常见的聚类算法,用于将一组数据分成几个类别。以下是使用Python编写的K-means代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
def kmeans(data, k, max_iterations=100):
# 初始化随机的k个中心点
centroids = initialize_centroids(data, k)
for _ in range(max_iterations):
# 将数据分配给最近的中心点
labels = assign_labels(data, centroids)
# 更新中心点的位置
new_centroids = update_centroids(data, labels, k)
# 如果中心点移动非常小,算法收敛
if np.allclose(centroids, new_centroids):
break
centroids = new_centroids
return labels, centroids
def initialize_centroids(data, k):
# 从数据中随机选择k个点作为初始中心点
indices = np.random.choice(len(data), k, replace=False)
centroids = data[indices]
return centroids
def assign_labels(data, centroids):
# 将每个数据点分配给最近的中心点
labels = np.argmin(np.linalg.norm(data[:, np.newaxis] - centroids, axis=-1), axis=1)
return labels
def update_centroids(data, labels, k):
# 计算每个类别的新中心点
new_centroids = np.array([data[labels == i].mean(axis=0) for i in range(k)])
return new_centroids
# 生成示例数据
np.random.seed(0)
data = np.concatenate([np.random.randn(100, 2) + [2, 2], np.random.randn(100, 2) + [-2, -2]])
# 调用K-means算法
labels, centroids = kmeans(data, k=2)
# 可视化结果
plt.scatter(data[:, 0], data[:, 1], c=labels)
plt.scatter(centroids[:, 0], centroids[:, 1], c='red', marker='x')
plt.show()
```
这段代码首先定义了一个`kmeans`函数,其中使用了三个辅助函数:`initialize_centroids`用于初始化k个随机的中心点,`assign_labels`用于将每个数据点分配给最近的中心点,`update_centroids`用于更新每个类别的新中心点。
然后,代码生成了一组示例数据,并调用了`kmeans`函数进行聚类。最后,通过使用Matplotlib库对数据点和中心点进行可视化展示。
相关推荐
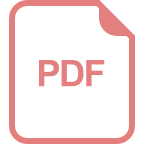
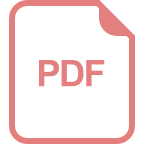














