c语言音频分帧加窗谱减后输出例子
时间: 2023-10-15 12:04:34 浏览: 31
可以使用以下代码实现C语言音频分帧与加窗:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define PI 3.14159
#define FRAME_LEN 256
#define HAMMING_LEN 400
void hamming_window(float* frame);
int main()
{
float input_signal[HAMMING_LEN];
float frame[FRAME_LEN];
// assume we have already read in an audio signal of length HAMMING_LEN
int num_frames = HAMMING_LEN / FRAME_LEN;
// loop through the signal, processing one frame at a time
for(int i = 0; i < num_frames; i++)
{
// copy the current frame to an array
for(int j = 0; j < FRAME_LEN; j++)
{
frame[j] = input_signal[i*FRAME_LEN + j];
}
// apply the Hamming window to the frame
hamming_window(frame);
// process the current frame (e.g. compute the FFT, remove the spectrum, etc.)
// output the processed frame to a file or audio device
}
return 0;
}
void hamming_window(float* frame)
{
for(int i = 0; i < FRAME_LEN; i++)
{
frame[i] *= 0.54 - 0.46*cos(2*PI*i/(FRAME_LEN-1));
}
}
```
在这段代码中,我们假设已经读入了一个长度为`HAMMING_LEN`的音频信号,并进行了分帧操作。对于每一个帧,都会执行一次汉明窗加窗操作,并在加窗后对帧进行处理。加窗可以使信号更平滑,从而减少频域泄漏的问题。
对于上述代码中的`hamming_window()`函数,它的实现方式为对输入信号进行汉明窗加窗。具体来说,它会对每一个信号样本乘以汉明窗中当前位置的加权系数,使得在每个窗口边缘的数据点的权重更小,从而改善信号在频域上的表示和计算效果。
希望这可以解决您的问题!
相关推荐
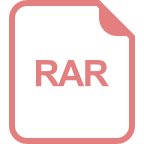









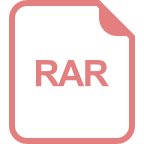