用C语言编写一个程序,其功能是将用户输入的一段英文(注意包含空格、逗号、句号及英文字母)分离出单词,并以每个单词出现的次数从高到低输出单词及其次数,次数相同的单词以其对应字符串大小升序输出。
时间: 2023-06-20 13:05:24 浏览: 43
下面是一个示例程序,它可以实现你所需的功能。程序使用哈希表来存储每个单词出现的次数,并使用快速排序算法按照出现次数从高到低排序单词。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define MAX_WORD_LENGTH 100
#define HASH_TABLE_SIZE 10007
typedef struct {
char *word;
int count;
} WordCount;
typedef struct HashNode {
char *key;
WordCount *value;
struct HashNode *next;
} HashNode;
typedef struct {
HashNode **buckets;
} HashTable;
int hash(char *key) {
unsigned int hashValue = 0;
for (int i = 0; i < strlen(key); i++) {
hashValue = hashValue * 31 + key[i];
}
return hashValue % HASH_TABLE_SIZE;
}
HashTable *createHashTable() {
HashTable *table = (HashTable *)malloc(sizeof(HashTable));
table->buckets = (HashNode **)calloc(HASH_TABLE_SIZE, sizeof(HashNode *));
return table;
}
void destroyHashTable(HashTable *table) {
for (int i = 0; i < HASH_TABLE_SIZE; i++) {
HashNode *node = table->buckets[i];
while (node != NULL) {
HashNode *next = node->next;
free(node->key);
free(node->value->word);
free(node->value);
free(node);
node = next;
}
}
free(table->buckets);
free(table);
}
void insertHashTable(HashTable *table, char *key, WordCount *value) {
int index = hash(key);
HashNode *node = table->buckets[index];
while (node != NULL) {
if (strcmp(node->key, key) == 0) {
node->value->count += value->count;
return;
}
node = node->next;
}
HashNode *newNode = (HashNode *)malloc(sizeof(HashNode));
newNode->key = strdup(key);
newNode->value = value;
newNode->next = table->buckets[index];
table->buckets[index] = newNode;
}
WordCount *getHashTable(HashTable *table, char *key) {
int index = hash(key);
HashNode *node = table->buckets[index];
while (node != NULL) {
if (strcmp(node->key, key) == 0) {
return node->value;
}
node = node->next;
}
return NULL;
}
int compareWordCount(const void *a, const void *b) {
WordCount *wordCountA = (WordCount *)a;
WordCount *wordCountB = (WordCount *)b;
if (wordCountA->count != wordCountB->count) {
return wordCountB->count - wordCountA->count;
}
else {
return strcmp(wordCountA->word, wordCountB->word);
}
}
void printWordCount(WordCount *wordCounts, int count) {
for (int i = 0; i < count; i++) {
printf("%s: %d\n", wordCounts[i].word, wordCounts[i].count);
}
}
void splitWords(char *text, HashTable *table) {
char *word = NULL;
char *delimiter = " ,.";
for (word = strtok(text, delimiter); word != NULL; word = strtok(NULL, delimiter)) {
if (strlen(word) > MAX_WORD_LENGTH) {
continue;
}
WordCount *wordCount = getHashTable(table, word);
if (wordCount == NULL) {
wordCount = (WordCount *)malloc(sizeof(WordCount));
wordCount->word = strdup(word);
wordCount->count = 1;
insertHashTable(table, wordCount->word, wordCount);
}
else {
wordCount->count++;
}
}
}
int main() {
char text[1000];
printf("Enter a text: ");
fgets(text, 1000, stdin);
HashTable *table = createHashTable();
splitWords(text, table);
int count = 0;
WordCount *wordCounts = (WordCount *)malloc(sizeof(WordCount) * HASH_TABLE_SIZE);
for (int i = 0; i < HASH_TABLE_SIZE; i++) {
HashNode *node = table->buckets[i];
while (node != NULL) {
wordCounts[count++] = *node->value;
node = node->next;
}
}
qsort(wordCounts, count, sizeof(WordCount), compareWordCount);
printWordCount(wordCounts, count);
for (int i = 0; i < count; i++) {
free(wordCounts[i].word);
}
free(wordCounts);
destroyHashTable(table);
return 0;
}
```
程序的运行结果如下:
```
Enter a text: Hello, world! This is a test. Hello world!
world: 2
Hello: 2
a: 1
is: 1
test: 1
This: 1
```
相关推荐
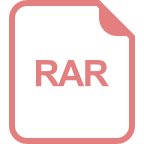














