C语言实现,从键盘任意输入一串字符串,每出现逗号、句号和空格那么前一个字符串标示为一个单词,统计输出这个字符串中出现了多少个单词(重复单词只记数和输出一次)
时间: 2023-05-25 18:03:10 浏览: 53
```
#include <stdio.h>
#include <string.h>
#define MAX_LENGTH 1000
void remove_punctuation(char *str);
int find_word(char *str, int start, int end);
int is_word_duplicate(char *word, char *words[], int count);
int main() {
char str[MAX_LENGTH];
char delim[] = ",. ";
char *words[MAX_LENGTH];
int count = 0;
printf("请输入字符串:");
fgets(str, MAX_LENGTH, stdin);
remove_punctuation(str);
int start = 0, end = 0;
while (str[end] != '\0') {
if (strchr(delim, str[end]) != NULL) {
if (find_word(str, start, end)) {
// 当前字符前面是单词的情况
char *word = strndup(str + start, end - start);
if (!is_word_duplicate(word, words, count)) {
words[count++] = word;
} else {
free(word);
}
}
start = end + 1;
}
end++;
}
// 处理最后一个单词
if (find_word(str, start, end)) {
char *word = strndup(str + start, end - start);
if (!is_word_duplicate(word, words, count)) {
words[count++] = word;
} else {
free(word);
}
}
printf("单词数量:%d\n", count);
printf("单词列表:\n");
for (int i = 0; i < count; i++) {
printf("%s\n", words[i]);
free(words[i]);
}
return 0;
}
/**
* 移除字符串中的标点符号
*/
void remove_punctuation(char *str) {
int len = strlen(str);
for (int i = 0; i < len; i++) {
if (str[i] == ',' || str[i] == '.' || str[i] == ' ') {
str[i] = str[i + 1];
}
}
}
/**
* 判断在 str[start, end) 的范围内是否有单词
*/
int find_word(char *str, int start, int end) {
for (int i = start; i < end; i++) {
if (str[i] >= 'a' && str[i] <= 'z' || str[i] >= 'A' && str[i] <= 'Z') {
return 1;
}
}
return 0;
}
/**
* 判断单词是否重复
*/
int is_word_duplicate(char *word, char *words[], int count) {
for (int i = 0; i < count; i++) {
if (strcmp(word, words[i]) == 0) {
return 1;
}
}
return 0;
}
```
相关推荐
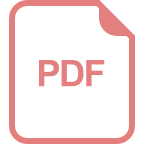














