file.readalllines需要关闭吗
时间: 2023-11-28 09:49:24 浏览: 117
在使用 `file.readlines()` 方法读取文件内容时,不需要显式地关闭文件。因为 `readlines()` 方法会自动打开文件并读取其中的内容,读取完成后也会自动关闭文件。但是为了保证代码的健壮性和可读性,我们通常还是建议在操作文件后手动关闭文件,以确保文件资源能够被及时释放。可以使用 `with` 语句来自动管理文件的打开和关闭,如下所示:
```python
with open('file.txt', 'r') as f:
lines = f.readlines()
# 在 with 语句块结束后,文件会自动关闭
```
在上面的例子中,当 `with` 语句块结束时,文件 `f` 会自动关闭。这样可以确保无论代码是否发生异常,文件都能被正确关闭。
相关问题
file.readalllines()需要关闭流吗 jdk8
`file.readAllLines()` 方法是 Java 8 中引入的,它返回一个包含文件所有行的列表。这个方法会自动关闭文件流,不需要手动关闭。这是因为在 Java 7 中引入了 `try-with-resources` 语句,可以在语句块结束时自动关闭资源。所以,当你使用 `readAllLines()` 方法时,文件流会自动关闭,不需要再手动关闭。以下是一个示例:
```
try {
List<String> lines = Files.readAllLines(Paths.get("filename.txt"));
for (String line : lines) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
```
在这个示例中,`Files.readAllLines()` 方法返回一个包含文件所有行的列表,然后我们遍历这个列表并打印每行。在 `try` 块结束时,文件流会自动关闭,不需要手动关闭。
public void importData() { try { List<String> courses = Files.readAllLines(Paths.get("data/courses.txt"), StandardCharsets.UTF_8); List<String> scores = Files.readAllLines(Paths.get("data/scores.txt"), StandardCharsets.UTF_8); List<String> ids = Files.readAllLines(Paths.get("data/credits.txt"), StandardCharsets.UTF_8); List<String> semesters = Files.readAllLines(Paths.get("data/semesters.txt"), StandardCharsets.UTF_8); List<String> courseTypes = Files.readAllLines(Paths.get("data/types.txt"), StandardCharsets.UTF_8); this.courses.clear(); for (int i = 0; i < courses.size(); i++) { String course = courses.get(i); String id = ids.get(i); String score = scores.get(i); String semester = semesters.get(i); String courseType = courseTypes.get(i); addData(course,id,score,semester,courseType); Course student = new Course(course, score,id, semester, courseType); this.courses.add(student); //updateDisplay(); } //updateDisplay(); JOptionPane.showMessageDialog(null, "Data has been imported"); } catch (IOException e) { JOptionPane.showMessageDialog(null, "An error occurred importing data"); } }优化这个代码
可以优化的几个方面:
1. 将重复的文件读取操作提取成一个方法,避免代码重复。
2. 使用 try-with-resources 语句来自动关闭资源,避免忘记关闭资源导致的资源泄漏。
3. 在 for 循环中,可以使用更简洁的 foreach 循环。
4. 在 for 循环中,不必要地创建了一个 Course 对象,可以直接调用 addData 方法添加数据。
优化后的代码如下:
```
public void importData() {
List<String> files = Arrays.asList("courses.txt", "scores.txt", "credits.txt", "semesters.txt", "types.txt");
List<List<String>> fileContents = new ArrayList<>();
for (String file : files) {
try (Stream<String> stream = Files.lines(Paths.get("data/" + file), StandardCharsets.UTF_8)) {
fileContents.add(stream.collect(Collectors.toList()));
} catch (IOException e) {
JOptionPane.showMessageDialog(null, "An error occurred importing data");
return;
}
}
this.courses.clear();
for (int i = 0; i < fileContents.get(0).size(); i++) {
String course = fileContents.get(0).get(i);
String id = fileContents.get(2).get(i);
String score = fileContents.get(1).get(i);
String semester = fileContents.get(3).get(i);
String courseType = fileContents.get(4).get(i);
addData(course, id, score, semester, courseType);
this.courses.add(new Course(course, score, id, semester, courseType));
}
JOptionPane.showMessageDialog(null, "Data has been imported");
}
```
阅读全文
相关推荐
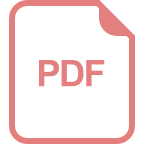
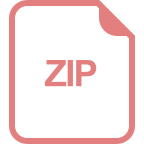

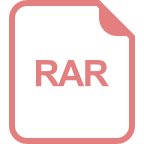
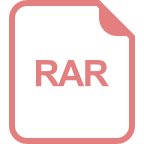
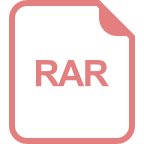
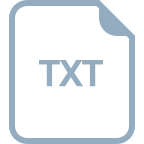
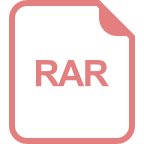
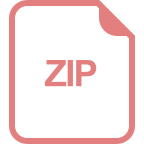
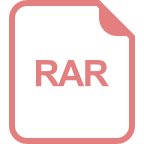
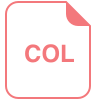
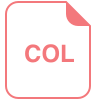



