linux小游戏源代码
时间: 2023-09-12 19:05:40 浏览: 82
### 回答1:
以下是一个简单的 Linux 小游戏的源代码,名为“猜数字游戏”:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main()
{
int number, guess, nguesses = 0;
srand(time(0));
number = rand() % 100 + 1; // 生成 1~100 随机数
do {
printf("猜一个数字 (1~100):");
scanf("%d", &guess);
nguesses++;
if (guess > number)
printf("太大了!\n");
else if (guess < number)
printf("太小了!\n");
else
printf("恭喜你,你猜对了,一共猜了 %d 次\n", nguesses);
} while (guess != number);
return 0;
}
```
该游戏会生成一个 1~100 的随机数,用户需要猜测这个数字是多少。如果猜错了,程序会提示用户数字是大了还是小了;如果猜对了,程序会输出用户猜了多少次,并退出游戏。
### 回答2:
Linux小游戏的源代码通常是用C或C++语言编写的,因为这两种语言在Linux平台上具有良好的兼容性。以下是一个简单的示例,是用C语言编写的一个命令行井字棋游戏的源代码:
```c
#include <stdio.h>
char board[3][3];
void initialize_board() {
int i, j;
for(i = 0; i < 3; i++) {
for(j = 0; j < 3; j++) {
board[i][j] = ' ';
}
}
}
void print_board() {
int i;
printf("---------\n");
for(i = 0; i < 3; i++) {
printf("| %c | %c | %c |\n", board[i][0], board[i][1], board[i][2]);
printf("---------\n");
}
}
int make_move(int x, int y, char player) {
if(x < 0 || x > 2 || y < 0 || y > 2) {
return 0;
}
if(board[x][y] != ' ') {
return 0;
}
board[x][y] = player;
return 1;
}
int check_winner(char player) {
int i;
for(i = 0; i < 3; i++) {
if(board[i][0] == player && board[i][1] == player && board[i][2] == player) {
return 1;
}
if(board[0][i] == player && board[1][i] == player && board[2][i] == player) {
return 1;
}
}
if(board[0][0] == player && board[1][1] == player && board[2][2] == player) {
return 1;
}
if(board[0][2] == player && board[1][1] == player && board[2][0] == player) {
return 1;
}
return 0;
}
int main() {
int x, y, player = 1;
initialize_board();
while(1) {
print_board();
printf("Player %d, enter your move (x y): ", player);
scanf("%d %d", &x, &y);
if(make_move(x, y, (player == 1) ? 'X' : 'O')) {
if(check_winner((player == 1) ? 'X' : 'O')) {
printf("Player %d wins!\n", player);
break;
}
player = (player == 1) ? 2 : 1;
} else {
printf("Invalid move. Try again.\n");
}
}
return 0;
}
```
这个源代码实现了一个基本的命令行井字棋游戏。玩家可以通过输入坐标在井字棋棋盘上放置自己的标记 'X' 或 'O',并在每次落子后判断是否有玩家获胜。游戏会不断循环直到有一名玩家获胜或者下棋平局。
### 回答3:
Linux是一个开源的操作系统,拥有庞大的软件生态系统。因此,在Linux上开发小游戏是一件很有乐趣的事情。
要提供一个小游戏的源代码,我选择了翻转棋(Reversi)游戏作为示例。以下是一个简单的Reversi游戏的源代码:
```python
# 导入所需模块
import numpy as np
# 初始化棋盘
board = np.zeros((8, 8))
board[3, 3] = board[4, 4] = 1
board[3, 4] = board[4, 3] = 2
# 定义棋盘显示函数
def display_board(board):
print(" ", end="")
for i in range(8):
print(chr(ord('a')+i), end=" ")
print()
for i in range(8):
print(str(i+1)+" ", end="")
for j in range(8):
if board[i, j] == 1:
print("●", end=" ")
elif board[i, j] == 2:
print("○", end=" ")
else:
print(" ", end=" ")
print()
# 定义翻转棋函数
def flip(board, row, col, player):
directions = [(0, 1), (0, -1), (1, 0), (-1, 0), (1, 1), (-1, -1), (1, -1), (-1, 1)]
for direction in directions:
r, c = row, col
r += direction[0]
c += direction[1]
if r >= 0 and r < 8 and c >= 0 and c < 8 and board[r, c] != player and board[r, c] != 0:
r += direction[0]
c += direction[1]
while r >= 0 and r < 8 and c >= 0 and c < 8 and board[r, c] != player and board[r, c] != 0:
r += direction[0]
c += direction[1]
if r >= 0 and r < 8 and c >= 0 and c < 8 and board[r, c] == player:
r -= direction[0]
c -= direction[1]
while board[r, c] != player:
board[r, c] = player
r -= direction[0]
c -= direction[1]
# 开始游戏
current_player = 1
display_board(board)
while True:
row = input("请玩家"+str(current_player)+"输入行数:")
col = input("请玩家"+str(current_player)+"输入列数:")
row = int(row)
col = ord(col) - ord('a')
if row >= 0 and row < 8 and col >= 0 and col < 8 and board[row, col] == 0:
flip(board, row, col, current_player)
current_player = 3 - current_player
display_board(board)
else:
print("无效的位置,请重新输入!")
```
这个源代码实现了一个简单的翻转棋游戏,玩家可以通过输入行数和列数来选择落子位置。游戏会自动翻转对方棋子,并显示当前棋盘状态。程序会不断循环直到游戏结束。
希望这个源代码可以帮助您开始在Linux上开发小游戏,并尝试在其中添加更多功能和改进。
相关推荐
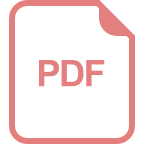
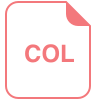
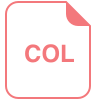
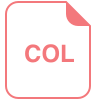











