用vue写一个论坛网页
时间: 2023-02-23 18:51:20 浏览: 165
要用Vue写一个论坛网页,需要遵循以下步骤:
1. 安装Vue CLI:在命令行中输入 "npm install -g @vue/cli" 安装Vue CLI。
2. 创建项目:使用 "vue create my-forum" 命令创建一个名为 "my-forum" 的新项目。
3. 安装依赖:在项目目录中使用命令 "npm install" 安装所需的依赖。
4. 构建 UI 组件:使用 Vue 组件,构建论坛页面的不同部分,例如帖子列表,帖子详情页面等。
5. 数据绑定:使用 Vue 的双向数据绑定功能,绑定视图与数据模型。
6. 路由配置:使用 Vue Router 在页面之间进行路由导航。
7. 部署:使用 "npm run build" 命令,将生成的文件部署到线上服务器,使得全世界都可以访问你的论坛网页。
这是一个大致的流程,你可以根据自己的需求添加或删除步骤。希望这些信息能帮助你开始写一个 Vue 论坛网页。
相关问题
用vue写一个主题网页
好的,下面是一个简单的主题网页的Vue代码示例:
```html
<template>
<div class="theme-page">
<header>
<h1>{{ title }}</h1>
<nav>
<ul>
<li v-for="item in menuItems" :key="item.id">
<a :href="item.link">{{ item.label }}</a>
</li>
</ul>
</nav>
</header>
<main>
<h2>Featured Articles</h2>
<section class="featured-articles">
<article v-for="article in featuredArticles" :key="article.id">
<h3>{{ article.title }}</h3>
<p>{{ article.summary }}</p>
<a :href="article.link">Read More</a>
</article>
</section>
<h2>Recent Articles</h2>
<section class="recent-articles">
<article v-for="article in recentArticles" :key="article.id">
<h3>{{ article.title }}</h3>
<p>{{ article.summary }}</p>
<a :href="article.link">Read More</a>
</article>
</section>
</main>
<footer>
<p>© 2021 My Theme Page</p>
</footer>
</div>
</template>
<script>
export default {
name: "ThemePage",
data() {
return {
title: "My Theme Page",
menuItems: [
{ id: 1, label: "Home", link: "#" },
{ id: 2, label: "About", link: "#" },
{ id: 3, label: "Contact", link: "#" },
],
featuredArticles: [
{
id: 1,
title: "Lorem Ipsum Dolor Sit Amet",
summary: "Lorem ipsum dolor sit amet, consectetur adipiscing elit.",
link: "#",
},
{
id: 2,
title: "Sed Do Eiusmod Tempor Incididunt",
summary: "Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.",
link: "#",
},
],
recentArticles: [
{
id: 1,
title: "Ut Enim Ad Minim Veniam",
summary: "Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.",
link: "#",
},
{
id: 2,
title: "Duis Aute Irure Dolor In Reprehenderit",
summary: "Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur.",
link: "#",
},
],
};
},
};
</script>
<style>
/* Add styles for the theme page here */
.theme-page {
font-family: sans-serif;
max-width: 800px;
margin: 0 auto;
padding: 20px;
}
header {
display: flex;
justify-content: space-between;
align-items: center;
}
nav ul {
display: flex;
list-style: none;
padding: 0;
}
nav li:not(:last-child) {
margin-right: 20px;
}
nav a {
text-decoration: none;
color: #333;
}
main {
margin-top: 40px;
}
h2 {
font-size: 24px;
margin-bottom: 20px;
}
section {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
}
article {
width: calc(50% - 10px);
margin-bottom: 20px;
padding: 20px;
background-color: #f5f5f5;
}
article h3 {
margin-top: 0;
}
article p {
margin-bottom: 10px;
}
article a {
text-decoration: underline;
}
</style>
```
上面的代码示例展示了一个简单的主题网页,包括页面标题、导航菜单、精选文章和最近文章等内容。你可以根据自己的需求进行修改和扩展。
用Vue2写一个网页计算器
好的,我可以回答这个问题。首先,你需要安装Vue2的开发环境,并创建一个Vue实例。然后,你可以使用Vue的模板语法来创建计算器的UI界面,包括数字按钮、运算符按钮和显示屏。接下来,你需要编写Vue的计算逻辑,将用户输入的数字和运算符进行计算,并将结果显示在屏幕上。最后,你可以添加一些样式和交互效果来提高用户体验。希望这可以帮助你开始编写一个Vue2的网页计算器。
相关推荐
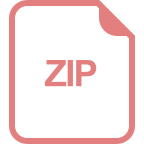
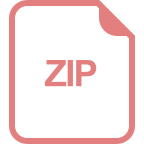












