实现将IP地址转换成一个整数
时间: 2024-05-01 21:16:22 浏览: 107
以下是Python的实现:
```python
def ip_to_int(ip):
"""
Convert an IP address to an integer.
"""
parts = ip.split('.')
res = 0
for i in range(4):
res += int(parts[i]) << (8 * (3 - i))
return res
```
这个函数将IP地址作为字符串输入,并返回一个整数。它将IP地址分成四个部分,并将每个部分转换为一个字节。然后,通过将每个字节向左移位,将它们组合成一个32位整数。
相关问题
实现将IP地址转换成一个整数python
可以使用Python内置的ipaddress模块来实现将IP地址转换成整数的功能。
示例代码:
```python
import ipaddress
ip_str = '192.168.1.1'
ip_int = int(ipaddress.IPv4Address(ip_str))
print(ip_int)
```
输出结果:
```
3232235777
```
解释:
将IP地址字符串`192.168.1.1`作为参数传入`IPv4Address`类中,创建一个IPv4Address对象,然后使用Python内置的`int()`函数将该对象转换成整数。最后输出结果为`3232235777`,即该IP地址对应的整数。
实现将IP地址转换成一个整数的完整代码
以下是将IP地址转换成一个整数的完整代码:
```
#include <stdio.h>
#include <string.h>
int ipToInt(char *ip) {
int a, b, c, d;
sscanf(ip, "%d.%d.%d.%d", &a, &b, &c, &d);
return (a << 24) | (b << 16) | (c << 8) | d;
}
int main() {
char ip[16];
printf("Please enter an IP address: ");
scanf("%s", ip);
int num = ipToInt(ip);
printf("The integer representation of %s is %d\n", ip, num);
return 0;
}
```
在这个代码中,`ipToInt`函数接收一个IP地址字符串,使用`sscanf`函数将其解析成四个整数,并使用位运算将它们转换成一个32位整数。`main`函数允许用户输入一个IP地址,并调用`ipToInt`函数将其转换为整数,并将结果输出到屏幕上。
阅读全文
相关推荐
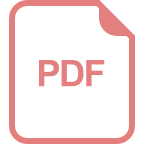
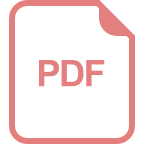







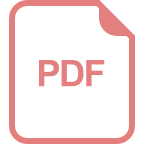
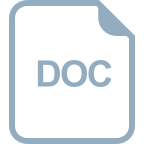
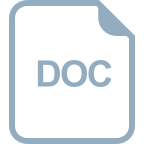
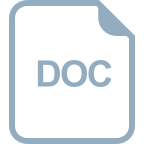
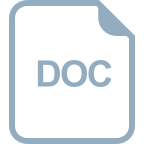
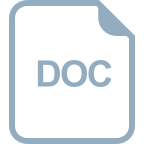