python 实现CNN算法
时间: 2023-10-16 15:11:02 浏览: 87
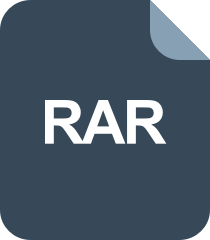
python实现的CNN代码

以下是一个简单的Python实现CNN算法的示例:
```python
import numpy as np
# 定义卷积函数
def convolve(image, kernel):
image_row, image_col = image.shape
kernel_row, kernel_col = kernel.shape
output = np.zeros((image_row - kernel_row + 1, image_col - kernel_col + 1))
for row in range(output.shape[0]):
for col in range(output.shape[1]):
output[row, col] = np.sum(image[row:row + kernel_row, col:col + kernel_col] * kernel)
return output
# 定义池化函数
def max_pooling(image, pool_size):
image_row, image_col = image.shape
pool_row, pool_col = pool_size
output = np.zeros((image_row // pool_row, image_col // pool_col))
for row in range(output.shape[0]):
for col in range(output.shape[1]):
output[row, col] = np.max(image[row * pool_row:(row + 1) * pool_row, col * pool_col:(col + 1) * pool_col])
return output
# 定义激活函数
def relu(image):
return np.maximum(image, 0)
# 定义全连接层函数
def fully_connected(input_data, weight, bias):
output = np.dot(input_data, weight) + bias
return output
# 定义CNN模型
class CNN:
def __init__(self, kernel_size, pool_size, num_filters, num_classes):
self.kernel_size = kernel_size
self.pool_size = pool_size
self.num_filters = num_filters
self.num_classes = num_classes
self.weights = []
self.biases = []
self.layers = []
def add_conv_layer(self, input_shape):
num_input_channels = input_shape[2]
kernel_shape = self.kernel_size + (num_input_channels, self.num_filters)
self.weights.append(np.random.randn(*kernel_shape))
self.biases.append(np.zeros((self.num_filters,)))
self.layers.append("conv")
def add_pooling_layer(self):
self.layers.append("pool")
def add_fully_connected_layer(self, input_shape):
input_size = input_shape[0] * input_shape[1] * input_shape[2]
self.weights.append(np.random.randn(input_size, self.num_classes))
self.biases.append(np.zeros((self.num_classes,)))
self.layers.append("fully_connected")
def forward(self, input_data):
output = input_data
for i in range(len(self.layers)):
if self.layers[i] == "conv":
output = convolve(output, self.weights[i]) + self.biases[i]
output = relu(output)
elif self.layers[i] == "pool":
output = max_pooling(output, self.pool_size)
elif self.layers[i] == "fully_connected":
output = output.reshape((output.shape[0], -1))
output = fully_connected(output, self.weights[i], self.biases[i])
return output
# 测试CNN模型
cnn = CNN(kernel_size=(3, 3), pool_size=(2, 2), num_filters=32, num_classes=10)
cnn.add_conv_layer(input_shape=(28, 28, 1))
cnn.add_pooling_layer()
cnn.add_fully_connected_layer(input_shape=(7, 7, 32))
input_data = np.random.randn(1, 28, 28, 1)
output = cnn.forward(input_data)
print(output)
```
在这个示例中,我们实现了一个简单的CNN模型,包括卷积层、池化层和全连接层。我们使用ReLU作为激活函数,并使用随机初始化权重和偏置项。在测试模型时,我们使用随机生成的28x28x1的输入数据,并输出模型的预测结果。
阅读全文
相关推荐
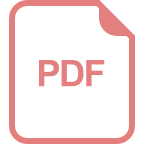
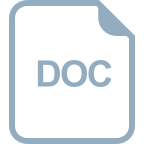
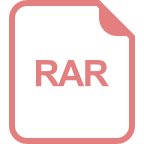
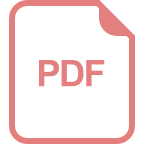
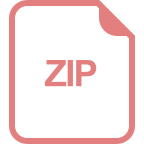
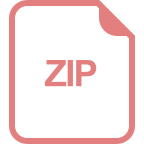
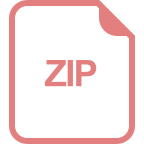
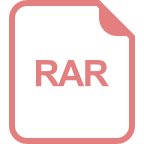
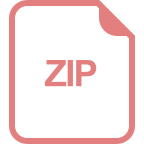
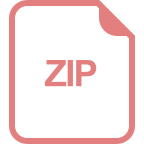
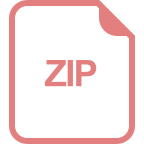
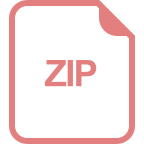
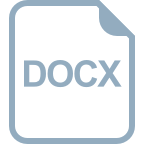
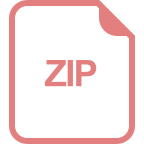
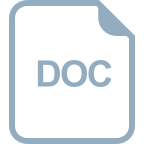
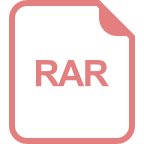