编写JSP程序,演示使用EL运算符进行基本运算。 实验步骤: (1)编写JSP程序el_operator.jsp; (2)查看程序运行效果;
时间: 2024-03-08 07:47:49 浏览: 87
下面是el_operator.jsp的代码,演示了使用EL运算符进行基本运算:
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>EL运算符示例</title>
</head>
<body>
<%-- 定义两个变量 --%>
<%
int a = 10;
int b = 5;
%>
<%-- 输出两个变量的值 --%>
<p>a 的值为:${a}</p>
<p>b 的值为:${b}</p>
<%-- 进行基本运算 --%>
<p>a + b 的值为:${a + b}</p>
<p>a - b 的值为:${a - b}</p>
<p>a * b 的值为:${a * b}</p>
<p>a / b 的值为:${a / b}</p>
<p>a % b 的值为:${a % b}</p>
</body>
</html>
```
在浏览器中访问el_operator.jsp,即可看到运行效果。页面会输出两个变量a和b的值,以及进行基本运算后的结果。其中,${}表示EL表达式,可以在JSP页面中使用它来获取变量的值,进行运算等操作。
相关问题
用jsp写加减乘除运算代码
在JSP (JavaServer Pages) 中编写加减乘除的简单运算代码,可以利用HTML表单让用户输入数值,然后通过Java脚本引擎(EL表达式或JSTL标签库)来处理计算。这里是一个简单的示例:
```jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<!DOCTYPE html>
<html>
<head>
<title>算数运算</title>
</head>
<body>
<form action="calculate.jsp" method="post">
<label for="num1">第一个数字:</label>
<input type="number" id="num1" name="num1"><br><br>
<label for="op">运算符 (+,-,*,/):</label>
<select id="op" name="op">
<option value="+">+</option>
<option value="-">-</option>
<option value="*">*</option>
<option value="/">/</option>
</select><br><br>
<input type="number" id="num2" name="num2">
<button type="submit">计算</button>
</form>
<%@ include file="calculator.jsp" %>
</body>
</html>
```
这是一个基本的HTML表单,用户输入两个数和选择运算符。`calculate.jsp` 表单提交后会包含引用`calculator.jsp` 文件,里面是计算逻辑:
**calculator.jsp**
```jsp
<%
String num1 = request.getParameter("num1");
String num2 = request.getParameter("num2");
String op = request.getParameter("op");
double result;
switch(op) {
case "+":
result = Double.parseDouble(num1) + Double.parseDouble(num2);
break;
case "-":
result = Double.parseDouble(num1) - Double.parseDouble(num2);
break;
case "*":
result = Double.parseDouble(num1) * Double.parseDouble(num2);
break;
case "/":
if(Double.parseDouble(num2) != 0) {
result = Double.parseDouble(num1) / Double.parseDouble(num2);
} else {
// 防止除以零错误
result = "Error! Division by zero is not allowed.";
}
break;
default:
result = "Invalid operator!";
break;
}
out.println("结果: " + result);
%>
```
在这个例子中,我们获取用户的输入,根据运算符进行相应的计算,并将结果显示在页面上。
JavaBean+Servlet+JSP实现复数的四则运算
好的,我可以为您提供一个 JavaBean+Servlet+JSP 实现复数的四则运算的示例代码。
首先,我们需要创建一个 JavaBean 类,用于表示复数。这个类应该包含两个属性,分别表示复数的实部和虚部。同时,这个类还应该提供一些方法,用于实现复数的四则运算。例如:
```java
public class ComplexNumber {
private double realPart; // 实部
private double imaginaryPart; // 虚部
public ComplexNumber(double realPart, double imaginaryPart) {
this.realPart = realPart;
this.imaginaryPart = imaginaryPart;
}
public double getRealPart() {
return realPart;
}
public void setRealPart(double realPart) {
this.realPart = realPart;
}
public double getImaginaryPart() {
return imaginaryPart;
}
public void setImaginaryPart(double imaginaryPart) {
this.imaginaryPart = imaginaryPart;
}
public ComplexNumber add(ComplexNumber other) {
double real = this.realPart + other.realPart;
double imaginary = this.imaginaryPart + other.imaginaryPart;
return new ComplexNumber(real, imaginary);
}
public ComplexNumber subtract(ComplexNumber other) {
double real = this.realPart - other.realPart;
double imaginary = this.imaginaryPart - other.imaginaryPart;
return new ComplexNumber(real, imaginary);
}
public ComplexNumber multiply(ComplexNumber other) {
double real = this.realPart * other.realPart - this.imaginaryPart * other.imaginaryPart;
double imaginary = this.realPart * other.imaginaryPart + this.imaginaryPart * other.realPart;
return new ComplexNumber(real, imaginary);
}
public ComplexNumber divide(ComplexNumber other) {
double denominator = other.realPart * other.realPart + other.imaginaryPart * other.imaginaryPart;
double real = (this.realPart * other.realPart + this.imaginaryPart * other.imaginaryPart) / denominator;
double imaginary = (this.imaginaryPart * other.realPart - this.realPart * other.imaginaryPart) / denominator;
return new ComplexNumber(real, imaginary);
}
}
```
接下来,我们需要创建一个 Servlet 类,用于处理用户请求。这个 Servlet 类应该根据用户输入的参数进行相应的运算,并将结果存储在 request 对象中,然后将请求转发到 JSP 页面。例如:
```java
@WebServlet("/complex-calculate")
public class ComplexCalculatorServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 获取用户输入的参数
double num1Real = Double.parseDouble(request.getParameter("num1-real"));
double num1Imaginary = Double.parseDouble(request.getParameter("num1-imaginary"));
double num2Real = Double.parseDouble(request.getParameter("num2-real"));
double num2Imaginary = Double.parseDouble(request.getParameter("num2-imaginary"));
String operator = request.getParameter("operator");
// 根据用户选择的运算符进行运算
ComplexNumber num1 = new ComplexNumber(num1Real, num1Imaginary);
ComplexNumber num2 = new ComplexNumber(num2Real, num2Imaginary);
ComplexNumber result = null;
switch(operator) {
case "+":
result = num1.add(num2);
break;
case "-":
result = num1.subtract(num2);
break;
case "*":
result = num1.multiply(num2);
break;
case "/":
result = num1.divide(num2);
break;
}
// 将结果存储在 request 对象中
request.setAttribute("num1", num1);
request.setAttribute("num2", num2);
request.setAttribute("operator", operator);
request.setAttribute("result", result);
// 转发请求到 JSP 页面
RequestDispatcher dispatcher = request.getRequestDispatcher("/complex-calculate.jsp");
dispatcher.forward(request, response);
}
}
```
最后,我们需要创建一个 JSP 页面,用于显示运算结果。这个 JSP 页面应该从 request 对象中获取计算结果,并将其显示在页面上。例如:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>复数四则运算</title>
</head>
<body>
<h1>复数四则运算</h1>
<form action="complex-calculate" method="GET">
<input type="text" name="num1-real"> + <input type="text" name="num1-imaginary"> i
<select name="operator">
<option value="+">+</option>
<option value="-">-</option>
<option value="*">*</option>
<option value="/">/</option>
</select>
<input type="text" name="num2-real"> + <input type="text" name="num2-imaginary"> i
<input type="submit" value="计算">
</form>
<c:if test="${not empty result}">
<p>${num1.realPart}+${num1.imaginaryPart}i ${operator} ${num2.realPart}+${num2.imaginaryPart}i = ${result.realPart}+${result.imaginaryPart}i</p>
</c:if>
</body>
</html>
```
在这个 JSP 页面中,我们使用 JSTL 标签库来判断是否有计算结果,如果有,就将结果显示在页面上。注意,我们在 JSP 页面中使用 EL 表达式来获取 JavaBean 对象的属性。
阅读全文
相关推荐
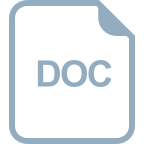
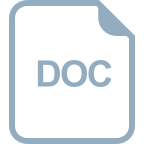
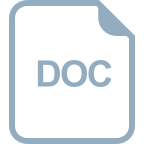
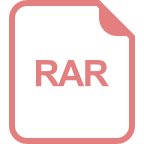
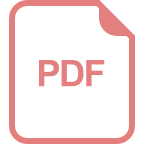
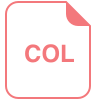
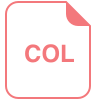
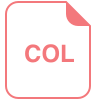


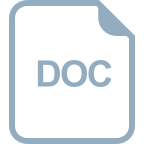
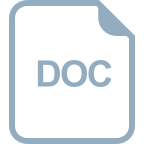
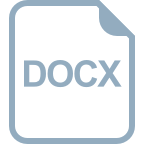
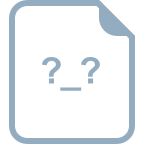