python对rosbag中的两组相机数据进行软同步并生成新的rosbag代码
时间: 2024-05-16 20:14:12 浏览: 99
要实现软同步两组相机数据并生成新的ROS bag文件,需要使用ROS中的一些工具和Python的一些库。具体步骤如下:
1. 安装ROS和Python库
首先需要安装ROS和相关的Python库,比如rospy、cv_bridge等。
2. 读取原始的ROS bag文件
使用ROS中的rosbag库读取原始的ROS bag文件,并提取出两组相机数据。
```python
import rosbag
bag = rosbag.Bag('original.bag')
cam1_msgs = []
cam2_msgs = []
for topic, msg, t in bag.read_messages(topics=['/cam1/image_raw', '/cam2/image_raw']):
if topic == '/cam1/image_raw':
cam1_msgs.append((msg, t.to_sec()))
elif topic == '/cam2/image_raw':
cam2_msgs.append((msg, t.to_sec()))
```
3. 实现软同步
实现软同步需要根据两组相机数据之间的时间戳进行插值。这里可以使用Python中的numpy库和scipy库来实现。
```python
import numpy as np
from scipy.interpolate import interp1d
# 将两组相机数据的时间戳取出来
cam1_times = [t for _, t in cam1_msgs]
cam2_times = [t for _, t in cam2_msgs]
# 将时间戳转化为相对时间
cam1_rel_times = np.array(cam1_times) - cam1_times[0]
cam2_rel_times = np.array(cam2_times) - cam2_times[0]
# 对两组相机数据的时间戳进行插值
f1 = interp1d(cam1_rel_times, cam1_msgs, axis=0, bounds_error=False)
f2 = interp1d(cam2_rel_times, cam2_msgs, axis=0, bounds_error=False)
# 以较短的时间戳为准进行插值
start_time = max(cam1_rel_times[0], cam2_rel_times[0])
end_time = min(cam1_rel_times[-1], cam2_rel_times[-1])
timestamps = np.linspace(start_time, end_time, int((end_time - start_time) / 0.1))
# 生成新的相机数据
new_cam1_msgs = [f1(t) for t in timestamps]
new_cam2_msgs = [f2(t) for t in timestamps]
```
4. 写入新的ROS bag文件
将生成的新的相机数据写入新的ROS bag文件中。
```python
new_bag = rosbag.Bag('synced.bag', 'w')
for msg, t in new_cam1_msgs:
new_bag.write('/cam1/image_raw', msg, rospy.Time.from_sec(t))
for msg, t in new_cam2_msgs:
new_bag.write('/cam2/image_raw', msg, rospy.Time.from_sec(t))
new_bag.close()
```
完整代码如下:
```python
import rosbag
import numpy as np
from scipy.interpolate import interp1d
bag = rosbag.Bag('original.bag')
cam1_msgs = []
cam2_msgs = []
for topic, msg, t in bag.read_messages(topics=['/cam1/image_raw', '/cam2/image_raw']):
if topic == '/cam1/image_raw':
cam1_msgs.append((msg, t.to_sec()))
elif topic == '/cam2/image_raw':
cam2_msgs.append((msg, t.to_sec()))
cam1_times = [t for _, t in cam1_msgs]
cam2_times = [t for _, t in cam2_msgs]
cam1_rel_times = np.array(cam1_times) - cam1_times[0]
cam2_rel_times = np.array(cam2_times) - cam2_times[0]
f1 = interp1d(cam1_rel_times, cam1_msgs, axis=0, bounds_error=False)
f2 = interp1d(cam2_rel_times, cam2_msgs, axis=0, bounds_error=False)
start_time = max(cam1_rel_times[0], cam2_rel_times[0])
end_time = min(cam1_rel_times[-1], cam2_rel_times[-1])
timestamps = np.linspace(start_time, end_time, int((end_time - start_time) / 0.1))
new_cam1_msgs = [f1(t) for t in timestamps]
new_cam2_msgs = [f2(t) for t in timestamps]
new_bag = rosbag.Bag('synced.bag', 'w')
for msg, t in new_cam1_msgs:
new_bag.write('/cam1/image_raw', msg, rospy.Time.from_sec(t))
for msg, t in new_cam2_msgs:
new_bag.write('/cam2/image_raw', msg, rospy.Time.from_sec(t))
new_bag.close()
```
阅读全文
相关推荐
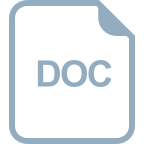
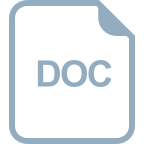
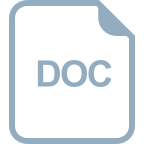
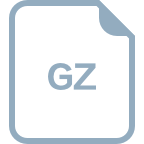
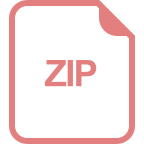
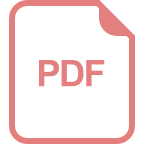
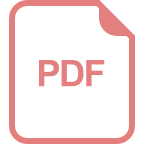
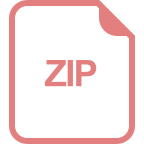
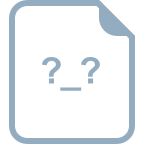
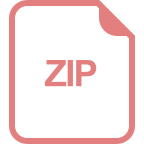
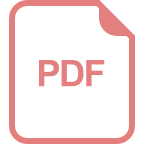
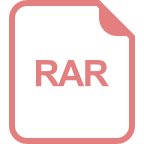
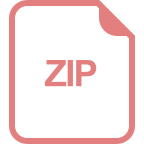
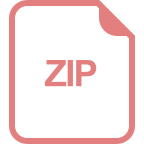
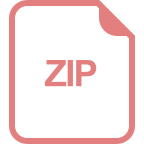