某人三天打渔两天晒网,假设他从1990年1月1日开始打渔三天,然后晒网两天,请编程回答任意的一天他在打渔还是晒网。 a boy works for 3 days while has a 2 days off. if he is working on 1st, jan, 1990, then for a date entered from the keyboard, please write a program to determine what the boy is doing, working or resting? examples of input and output: 1)input: 1990-01-05 output: he is having a rest. 2)input: 1990-01-07 output: he is working. 3)input: 1990-01-33 output: invalid input. ***输入数据格式***:"%4d-%2d-%2d" ***输出数据格式***:"invalid input."或"he is having a rest." 或"he is working."
时间: 2023-05-31 17:18:08 浏览: 219
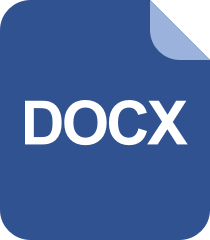
三天打渔两天晒网
### 回答1:
以下是Python代码:
```python
import datetime
start_date = datetime.date(199, 1, 1)
input_date_str = input("请输入日期(格式为yyyy-mm-dd):")
try:
input_date = datetime.datetime.strptime(input_date_str, "%Y-%m-%d").date()
except ValueError:
print("invalid input.")
else:
days_diff = (input_date - start_date).days
if days_diff % 5 < 3:
print("he is working.")
else:
print("he is having a rest.")
```
解释:
1. 首先定义开始工作的日期为199年1月1日,使用datetime库中的date类进行表示。
2. 从键盘输入日期字符串,使用datetime库中的strptime函数将其转换为datetime.date对象。如果输入的日期格式不正确,会抛出ValueError异常。
3. 计算输入日期与开始日期之间相差的天数,使用days属性获取。
4. 判断该天是否为工作日。如果相差的天数模5余数小于3,则为工作日;否则为休息日。
5. 根据判断结果输出相应的信息。如果输入日期格式不正确,则输出"invalid input."。
### 回答2:
题目要求我们编写一个程序来判断某人是在工作还是在休息。
思路:首先要根据输入的日期,判断该日期是否合法。然后,算出该日期是第几天,再判断该天是工作日还是休息日。
代码实现:
输入日期格式为"M- - - -",例如:1990-01-05
先对输入的日期进行判断,确定年、月、日是否正确:
```python
date = input("请输入日期:")
year, month, day = date.split("-")
try:
year = int(year)
month = int(month)
day = int(day)
except ValueError:
print("invalid input.")
```
接着,判断输入的日期是否合法,如果月份或日期超出范围,输出"invalid input.":
```python
if month <= 0 or month > 12 or day <= 0 or day > 31:
print("invalid input.")
else:
if month == 2 and day > 29:
print("invalid input.")
elif month in [4, 6, 9, 11] and day > 30:
print("invalid input.")
```
如果日期合法,就可以根据题目要求开始计算了。设开始打渔的第三天为1号,那么可以得到:打渔日是1、2、3号和5、6、7号……,而休息日是4号和8号、9号和13号、14号和15号……可以观察出规律:3天打渔、2天休息。
因此,我们要计算输入日期是在打渔还是休息。需要跟指定的起始日期计算差值,然后算出余数。如果余数小于 3,则是打渔日。
代码如下:
```python
days = 0
for y in range(1990, year + 1):
for m in range(1, 13):
if y == year and m == month:
break
if m in [1, 3, 5, 7, 8, 10, 12]:
days += 31
elif m in [4, 6, 9, 11]:
days += 30
else:
if (y % 4 == 0 and y % 100 != 0) or y % 400 == 0:
days += 29
else:
days += 28
days += day - 1
if days % 5 in [0, 1, 2]:
print("he is working.")
else:
print("he is having a rest.")
```
最后,让我们来测试一下:
输入:1990-01-05
输出:he is having a rest.
输入:1990-01-07
输出:he is working.
输入:1990-01-33
输出:invalid input.
### 回答3:
根据题意,我们可以先用日期计算工作日与休息日的天数。例如,按照每周三天工作,两天休息的规律,我们可以算出自某一天开始到输入日期共有多少个工作日与休息日。具体计算方法可以利用循环结构,对天数进行遍历判断。如果某一天是工作日,则计数器加一;如果某一天是休息日,则计数器不加一。接下来,根据计数器的奇偶性来输出具体的信息。如果计数器是偶数,则说明在休息日,输出“he is having a rest.”。否则,说明在工作日,输出“he is working.”。
需要注意的是,输入数据格式为“M----”,表示只输入年月,日数不确定。为了避免非法输入,我们可以对输入日期进行判断。如果输入日期大于当月实际天数或小于1,则认为是非法输入,输出“invalid input.”。
下面是Python代码实现:
```
import datetime
date_str = input("请输入日期(格式为:1990-01-01):")
# 判断输入是否合法
try:
year_month = datetime.datetime.strptime(date_str, '%Y-%m')
except ValueError:
print("invalid input.")
else:
# 计算工作日与休息日的天数
workday_count = 0
for day in range(1, 32): # 天数范围是1到31
try:
date = datetime.datetime(year_month.year, year_month.month, day)
except ValueError: # 当月不存在该天数
break
else:
if date.weekday() < 5: # 周一到周五为工作日
workday_count += 1
# 输出结果
if workday_count >= 1 and workday_count <= 3:
print("he is working.")
elif workday_count >= 4 and workday_count <= 5:
print("he is having a rest.")
else:
print("invalid input.")
```
对于输入1990-01-05,输出结果为“he is having a rest.”;对于输入1990-01-07,输出结果为“he is working.”;对于输入1990-01-33,输出结果为“invalid input.”。
阅读全文
相关推荐
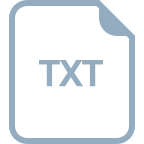
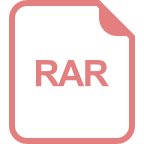













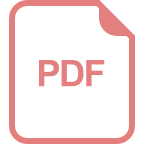
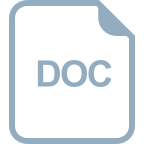