写出java实现发表文章的功能代码 流程: 1:用户在首页点击发表文章的超链接来到发表文章页面 2:在页面输入文章标题和文章内容并点击发表按钮 3:服务端将该文章保存后响应发表结果页面(成功或失败)
时间: 2024-02-06 18:04:44 浏览: 136
好的,以下是Java实现发表文章功能的代码:
在静态下新建对应的页面:
writeArticle.html
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>发表文章</title>
</head>
<body>
<h1>发表文章</h1>
<form action="/writeArticle" method="post">
<label for="title">标题:</label>
<input type="text" id="title" name="title" required>
<br>
<label for="content">内容:</label>
<textarea id="content" name="content" rows="10" cols="50" required></textarea>
<br>
<label for="author">作者:</label>
<input type="text" id="author" name="author" required>
<br>
<input type="submit" value="发表">
</form>
</body>
</html>
```
article_success.html
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>发表成功</title>
</head>
<body>
<h1>发表成功!</h1>
<p><a href="/">返回首页</a></p>
</body>
</html>
```
article_fail.html
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>发表失败</title>
</head>
<body>
<h1>发表失败!</h1>
<p><a href="/">返回首页</a></p>
</body>
</html>
```
在controller包下新建处理文章相关的业务类ArticleController,并定义处理发表文章的方法writeArticle(HttpServletRequest request,HttpServletResponse response)。需要使用@Controller注解和@RequestMapping注解来指定请求的URL和请求方法。
ArticleController.java
```java
package com.example.controller;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import com.example.entity.Article;
@Controller
public class ArticleController {
@RequestMapping(value = "/writeArticle", method = RequestMethod.POST)
public String writeArticle(HttpServletRequest request, HttpServletResponse response) {
String title = request.getParameter("title");
String content = request.getParameter("content");
String author = request.getParameter("author");
if (title == null || title.trim().isEmpty() || content == null || content.trim().isEmpty()) {
return "article_fail";
}
Article article = new Article(title, content, author);
try {
FileOutputStream fos = new FileOutputStream("article/" + title + ".obj");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(article);
oos.close();
fos.close();
} catch(IOException e) {
e.printStackTrace();
return "article_fail";
}
return "article_success";
}
}
```
在entity包下新建表示文章的对象Article并实现序列化接口。
Article.java
```java
package com.example.entity;
import java.io.Serializable;
public class Article implements Serializable {
private static final long serialVersionUID = 1L;
private String title;
private String content;
private String author;
public Article(String title, String content, String author) {
this.title = title;
this.content = content;
this.author = author;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
}
```
以上就是Java实现发表文章功能的代码。
阅读全文
相关推荐
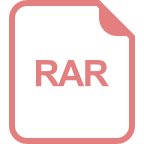
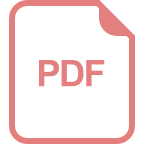
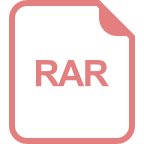
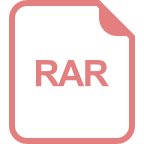
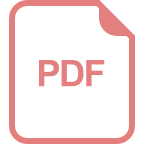
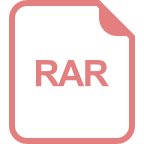
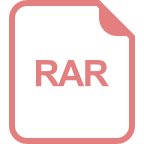
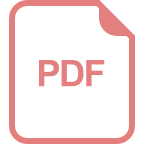
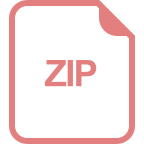
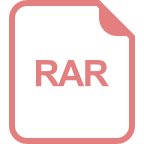
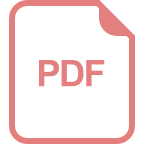
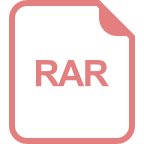
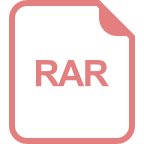
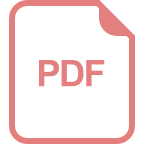
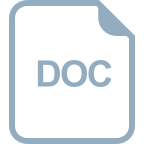