analyse the document
时间: 2024-11-09 22:20:53 浏览: 20
### Document Analysis: Week 7 Exercises
#### Overview
The document contains a series of exercises focused on Object-Oriented Programming (OOP) concepts in Python. The exercises cover creating classes, initializing objects, modifying object properties, and manipulating dictionaries.
#### Key Exercises and Concepts
1. **Creating a Class and Setting Variables**
- **Task**: Create a class `MyClass` with a variable `x` set to 2.
- **Example**:
```python
class MyClass:
x = 2
```
2. **Creating an Object and Accessing Variables**
- **Task**: Create an object `p1` of `MyClass` and print the value of `x`.
- **Example**:
```python
p1 = MyClass()
print(p1.x)
```
3. **Initializing Objects with `__init__` Method**
- **Task**: Define a class `Person` with an `__init__` method to initialize `name` and `age`.
- **Example**:
```python
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
```
4. **Using Object Methods to Print Values**
- **Task**: Create a class `Brother` with an `__init__` method and print `name` and `age` using object methods.
- **Example**:
```python
class Brother:
def __init__(self, name, age):
self.name = name
self.age = age
def print_info(self):
print(f"Name: {self.name}, Age: {self.age}")
b1 = Brother("John", 25)
b1.print_info()
```
5. **Printing Within the Function Using Concatenation**
- **Task**: Similar to the previous task, but print within the `__init__` method.
- **Example**:
```python
class Brother:
def __init__(self, name, age):
self.name = name
self.age = age
print(f"Name: {self.name}, Age: {self.age}")
b1 = Brother("John", 25)
```
6. **Renaming `self` Parameter**
- **Task**: Rename `self` to another variable name in the `__init__` method.
- **Example**:
```python
class Brother:
def __init__(this, name, age):
this.name = name
this.age = age
print(f"Name: {this.name}, Age: {this.age}")
b1 = Brother("John", 25)
```
7. **Modifying Object Properties**
- **Task**: Modify an object property after initialization.
- **Example**:
```python
b1.age = 30
print(b1.age)
```
8. **Deleting Object Properties**
- **Task**: Delete an object property using the `del` keyword.
- **Example**:
```python
del b1.age
```
9. **Deleting Objects**
- **Task**: Delete the entire object using the `del` keyword.
- **Example**:
```python
del b1
```
10. **Dictionary Manipulation**
- **Task**: Use the `get` function to access a dictionary value.
- **Example**:
```python
guitar = {
"brand": "Gibson",
"model": "Les Paul",
"year": 1963
}
print(guitar.get("model"))
```
- **Task**: Change a dictionary value.
- **Example**:
```python
guitar["year"] = 1968
```
- **Task**: Add a new key-value pair to the dictionary.
- **Example**:
```python
guitar["colour"] = "red"
```
- **Task**: Remove a key-value pair from the dictionary using the `pop` method.
- **Example**:
```python
guitar.pop("model")
```
#### Summary
The document provides a comprehensive set of exercises to practice fundamental OOP concepts in Python, including class creation, object initialization, property manipulation, and dictionary operations. Each exercise builds upon the previous ones, gradually increasing in complexity. This structured approach helps reinforce understanding and application of OOP principles.
阅读全文
相关推荐
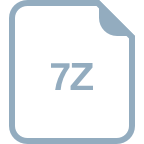
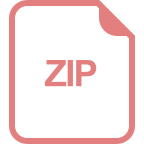
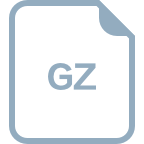












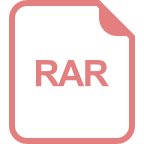
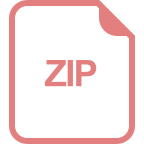
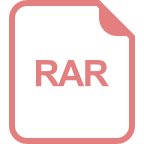