求解兵棋推演中最短路径算法python实现
时间: 2024-12-16 21:09:12 浏览: 8
在兵棋推演中,最短路径问题通常是指如何找到从初始位置到目标位置的最小步数或时间消耗。这可以使用图论中的算法来解决,比如Dijkstra算法(适用于带权重的边)或A*搜索算法(更适合于寻找启发式路径)。这里我会简要介绍如何用Python实现这两种方法。
**1. Dijkstra算法**
首先,你需要一个表示图的数据结构,如邻接矩阵或邻接表。然后,你可以按照以下步骤实现:
```python
import heapq
def dijkstra(graph, start):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
priority_queue = [(0, start)]
while priority_queue:
current_distance, current_node = heapq.heappop(priority_queue)
if current_distance > distances[current_node]:
continue # 如果已经找到更短路径,则忽略
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(priority_queue, (distance, neighbor))
return distances
# 使用时传入你的兵棋格子图(每个节点代表一个位置,邻接字典存储相邻位置及其代价)
```
**2. A*搜索算法**
A*算法结合了迪杰斯特拉的局部最优性和宽度优先搜索的全局信息。它需要一个启发函数来估计从当前节点到目标的“最佳”路径长度。具体实现如下:
```python
import heapq
def heuristic(a, b): # 假设曼哈顿距离或欧几里得距离作为启发函数
dx, dy = a[0] - b[0], a[1] - b[1]
return abs(dx) + abs(dy)
def a_star(graph, start, goal):
open_set = [(heuristic(start, goal), start)]
came_from = {}
cost_so_far = {start: 0}
while open_set:
_, current = heapq.heappop(open_set)
if current == goal:
break
for next_node, weight in graph[current].items():
new_cost = cost_so_far[current] + weight
if next_node not in cost_so_far or new_cost < cost_so_far[next_node]:
cost_so_far[next_node] = new_cost
priority = new_cost + heuristic(next_node, goal)
heapq.heappush(open_set, (priority, next_node))
came_from[next_node] = current
return came_from, cost_so_far
# 使用时同样传入图、起点和终点
```
阅读全文
相关推荐
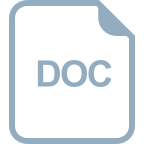
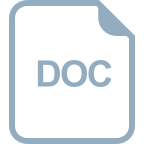
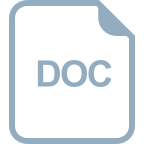

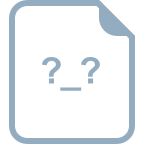
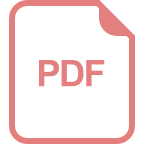
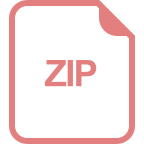
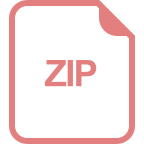
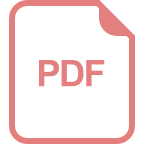
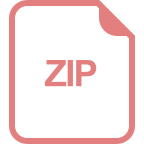
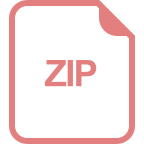
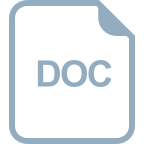
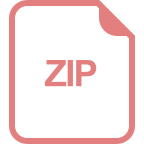
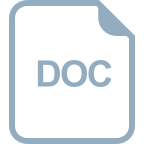
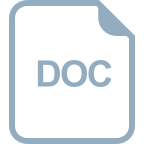

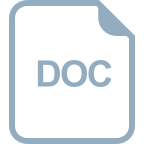
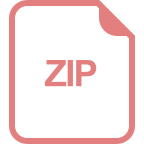
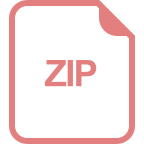