写一个使用esp8266开启热点,通过网页控制红外模块发射与接收信号,并且反馈到网页上的代码
时间: 2024-05-07 08:19:47 浏览: 135
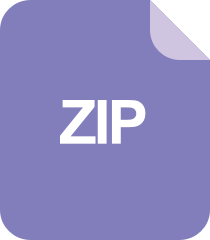
基于ESP8266的红外遥控器.zip
下面是一个使用ESP8266开启热点的示例代码。其中,热点名称为"InfraredController",密码为"12345678"。
```c++
#include <ESP8266WiFi.h>
const char* ssid = "InfraredController";
const char* password = "12345678";
void setup() {
Serial.begin(9600);
WiFi.softAP(ssid, password);
IPAddress myIP = WiFi.softAPIP();
Serial.print("AP IP address: ");
Serial.println(myIP);
}
void loop() {
// 等待连接
}
```
接下来,我们需要添加控制红外模块的代码。我们可以使用NEC红外协议。下面是一个使用IRremoteESP8266库的示例代码。
```c++
#include <ESP8266WiFi.h>
#include <IRremoteESP8266.h>
#include <IRsend.h>
#include <IRrecv.h>
#include <IRutils.h>
const char* ssid = "InfraredController";
const char* password = "12345678";
#define IR_SEND_PIN 4
#define IR_RECV_PIN 5
IRsend irsend(IR_SEND_PIN);
IRrecv irrecv(IR_RECV_PIN);
decode_results results;
void setup() {
Serial.begin(9600);
WiFi.softAP(ssid, password);
IPAddress myIP = WiFi.softAPIP();
Serial.print("AP IP address: ");
Serial.println(myIP);
irrecv.enableIRIn();
}
void loop() {
if (irrecv.decode(&results)) {
Serial.println(results.value, HEX);
irrecv.resume(); // 接收下一个值
// 发射红外信号
irsend.sendNEC(results.value, 32);
}
}
```
最后,我们需要将红外信号编码发送到网页上。我们可以使用ESPAsyncWebServer库来实现这个功能。下面是完整的代码:
```c++
#include <ESP8266WiFi.h>
#include <IRremoteESP8266.h>
#include <IRsend.h>
#include <IRrecv.h>
#include <IRutils.h>
#include <ESPAsyncWebServer.h>
const char* ssid = "InfraredController";
const char* password = "12345678";
#define IR_SEND_PIN 4
#define IR_RECV_PIN 5
IRsend irsend(IR_SEND_PIN);
IRrecv irrecv(IR_RECV_PIN);
decode_results results;
AsyncWebServer server(80);
void setup() {
Serial.begin(9600);
WiFi.softAP(ssid, password);
IPAddress myIP = WiFi.softAPIP();
Serial.print("AP IP address: ");
Serial.println(myIP);
irrecv.enableIRIn();
server.on("/", HTTP_GET, [](AsyncWebServerRequest *request){
String html = "<html><body><h1>红外控制器</h1><form method='post'><input type='text' name='code' placeholder='输入红外信号代码'><br><br><button type='submit'>发送</button></form></body></html>";
request->send(200, "text/html", html);
});
server.on("/", HTTP_POST, [](AsyncWebServerRequest *request){
String code = request->getParam("code")->value();
irsend.sendNEC(strtol(code.c_str(), NULL, 16), 32);
request->send(200, "text/plain", "OK");
});
server.begin();
}
void loop() {
if (irrecv.decode(&results)) {
Serial.println(results.value, HEX);
irrecv.resume(); // 接收下一个值
}
}
```
在浏览器中访问ESP8266的IP地址,即可打开控制页面。在页面中输入红外信号代码,点击发送按钮即可控制红外模块发射信号。同时,ESP8266也会接收从红外模块接收到的信号,并将其输出到串口中。
阅读全文
相关推荐
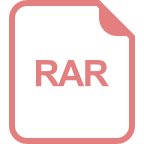

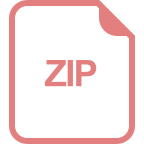
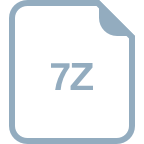
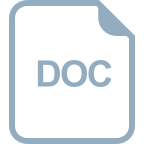
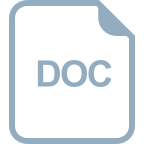
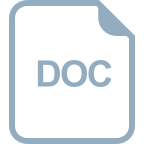
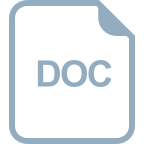
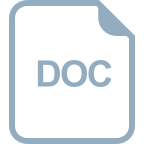
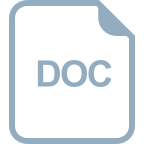





