python for else
时间: 2024-05-20 18:09:51 浏览: 159
Python的for循环中可以使用else语句,else语句会在循环正常执行完毕后执行。如果在循环中使用了break语句,那么else语句将不会被执行。
下面是一个简单的例子,使用for-else语句来查找素数:
```
for num in range(10, 20):
for i in range(2, num):
if num % i == 0:
print(num, "不是素数")
break
else:
print(num, "是素数")
```
在这个例子中,我们使用了两个for循环,外层循环枚举数字范围,内层循环用来判断一个数字是否是素数。如果内层循环中找到了一个因子,那么就会执行break语句跳出内层循环,不再进行后续的判断。如果内层循环正常执行完毕,也就是没有找到因子,那么就会执行else语句,输出该数字是素数。
相关问题
银行家算法 python
银行家算法是一种死锁避免算法,它可以确保在分配资源时不会发生死锁。下面是一个简单的Python实现:
```python
# 定义银行家算法类
class BankerAlgorithm:
def __init__(self, available, max, allocation):
self.available = available # 可用资源向量
self.max = max # 最大需求矩阵
self.allocation = allocation # 已分配矩阵
self.need = max - allocation # 需求矩阵
self.safe_sequence = [] # 安全序列
# 检查是否存在安全序列
def is_safe(self):
work = self.available.copy()
finish = [False] * len(self.max)
while False in finish:
found = False
for i in range(len(self.max)):
if not finish[i] and all(self.need[i] <= work):
work += self.allocation[i]
finish[i] = True
self.safe_sequence.append(i)
found = True
if not found:
return False
return True
# 分配资源
def allocate(self, process, request):
if all(request <= self.need[process]) and all(request <= self.available):
self.available -= request
self.allocation[process] += request
self.need[process] -= request
if self.is_safe():
return True
else:
self.available += request
self.allocation[process] -= request
self.need[process] += request
return False
else:
return False
```
使用示例:
```python
# 初始化银行家算法类
available = [3, 3, 2]
max = [[7, 5, 3], [3, 2, 2], [9, 0, 2], [2, 2, 2], [4, 3, 3]]
allocation = [[0, 1, 0], [2, 0, 0], [3, 0, 2], [2, 1, 1], [0, 0, 2]]
ba = BankerAlgorithm(available, max, allocation)
# 检查是否存在安全序列
if ba.is_safe():
print("存在安全序列:", ba.safe_sequence)
else:
print("不存在安全序列")
# 分配资源
if ba.allocate(1, [1, 0, 2]):
print("分配成功")
else:
print("分配失败")
```
python鸡兔同笼
鸡兔同笼是一个经典的数学问题,也可以用Python来解决。
假设有n个头和m个脚,问有多少只鸡和兔子?
假设鸡的数量为x,兔子的数量为y,则有以下两个方程式:
```
x + y = n
2x + 4y = m
```
我们可以用Python来解这个方程组,代码如下:
```python
def solve(n, m):
for x in range(n+1):
y = n - x
if 2*x + 4*y == m:
return x, y
return None
n = 10 # 头的数量
m = 28 # 脚的数量
result = solve(n, m)
if result:
print("鸡的数量为:%d,兔子的数量为:%d" % result)
else:
print("无解")
```
运行结果为:
```
鸡的数量为:6,兔子的数量为:4
```
说明有6只鸡和4只兔子。
阅读全文
相关推荐
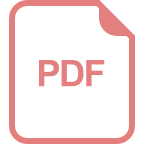
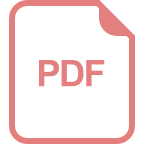
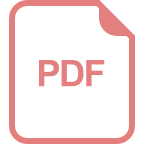


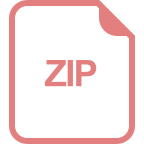










