倒立摆小游戏全过程代码
时间: 2024-06-13 18:02:10 浏览: 16
倒立摆(pendulum)是一个经典的物理模拟游戏,通常用于展示动态系统的复杂行为。在许多编程语言中,你可以使用基本的数学模型和图形库来实现。以下是一个简单的Python代码示例,使用`pygame`库来创建一个倒立摆游戏的基本框架:
```python
import pygame
import math
# 初始化Pygame
pygame.init()
# 设置窗口参数
window_size = (800, 600)
fps = 60
clock = pygame.time.Clock()
screen = pygame.display.set_mode(window_size)
pygame.display.set_caption("Pendulum Game")
# 倒立摆参数
length = 50
mass = 1
g = 9.8
angle_init = math.radians(30)
# 创建物理模拟类
class Pendulum:
def __init__(self, x, y, angle):
self.x = x
self.y = y
self.angle = angle
self.speed = 0
def update(self, dt):
self.speed += (-g * length * math.sin(self.angle)) * dt
self.angle += self.speed * dt
self.x = self.x + length * math.cos(self.angle) * dt
self.y = self.y + length * math.sin(self.angle) * dt
pendulum = Pendulum(window_size // 2, window_size - 50, angle_init)
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
# 清除屏幕
screen.fill((0, 0, 0))
# 绘制摆锤
pygame.draw.line(screen, (255, 255, 255), (pendulum.x, pendulum.y), (pendulum.x + length * math.cos(pendulum.angle), pendulum.y + length * math.sin(pendulum.angle)), 5)
# 更新并绘制摆动
pendulum.update(1 / fps)
# 更新屏幕
pygame.display.flip()
clock.tick(fps)
```
这个代码只是一个非常基础的版本,它没有处理碰撞检测、用户输入控制摆动角度或增加物理模拟的复杂性。要完整实现一个倒立摆游戏,你可能需要扩展代码来添加这些功能,并使用更复杂的图形渲染和动画。
相关推荐
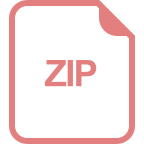
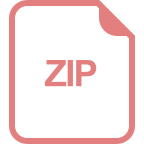
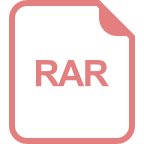
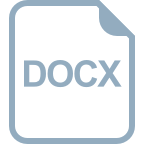
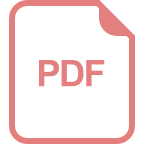
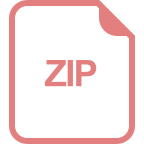
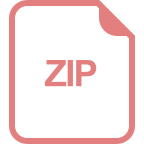
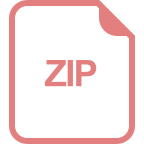