Python tkinter生成四则运算式,并判断回答的对错,统计对错数量
时间: 2024-09-20 15:17:59 浏览: 29
在Python中,我们可以利用Tkinter库创建一个简单的图形用户界面(GUI),结合基本的数学运算规则和条件语句来实现四则运算题目的生成并检查用户的输入是否正确。以下是简要步骤:
1. 首先,导入必要的模块:
```python
import tkinter as tk
from random import randint
```
2. 设计UI界面,包括两个输入框用于用户输入数字,一个用于显示题目,以及一个按钮用于提交答案:
```python
root = tk.Tk()
entry_1 = tk.Entry(root)
entry_2 = tk.Entry(root)
display = tk.Label(root)
button = tk.Button(root, text="计算")
```
3. 定义函数来生成随机算术表达式,检查答案,并更新UI状态:
```python
def generate_and_check():
operator = ['+', '-', '*', '/']
num1 = randint(1, 100)
num2 = randint(1, 100)
# 生成随机运算符和显示题目
operator_choice = operator[randint(0, len(operator) - 1)]
display_text = f"{num1} {operator_choice} {num2}"
display.config(text=display_text)
# 用户计算并输入答案
user_answer = int(entry_1.get()) + int(entry_2.get())
# 判断答案并更新结果
correct_answer = eval(f"{num1} {operator_choice} {num2}")
if user_answer == correct_answer:
result_text = "正确"
else:
result_text = "错误"
result_label = tk.Label(root, text=f"答案:{result_text}", font=("Arial", 14))
result_label.pack()
# 绑定按钮点击事件
button.config(command=generate_and_check)
```
4. 添加其他布局和关闭窗口的设置:
```python
entry_1.pack()
entry_2.pack()
button.pack(pady=10)
root.mainloop()
```
相关推荐
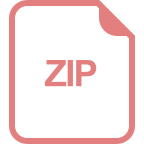
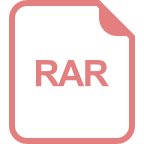
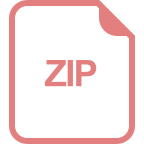










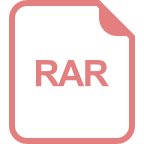
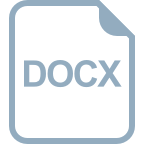
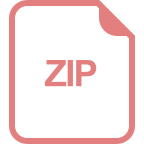
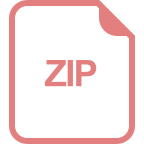
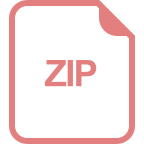