写一个摄影测量中空间后方交会c#程序
时间: 2024-10-21 07:02:15 浏览: 20
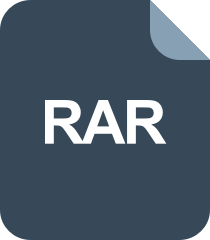
摄影测量_单张像片的空间后方交会(C# windows form)
在C#中编写一个摄影测量的空间后方交会(空间后方交汇法,也称为后方投影)算法涉及到地理信息系统(GIS)编程和三维几何计算。这是一个基本的步骤概述:
```csharp
using System;
using System.Collections.Generic;
using MathNet.Numerics.LinearAlgebra;
namespace Photogrammetry
{
public class SpatialBackProjection
{
// 真北方向向量
private Vector3D trueNorthDirection;
// 摄影机矩阵
private Matrix<double> cameraMatrix;
// 后方交会数据(如像点坐标、控制点坐标)
private List<XYZPoint> imagePoints;
private List<XYZPoint> controlPoints;
public void PerformBackProjection()
{
if (imagePoints.Count != controlPoints.Count)
throw new ArgumentException("Image points and control points must have the same number of elements.");
// 初始化线性系统
int n = imagePoints.Count;
double[,] system = new double[n, 4];
double[] unknowns = new double[4]; // 包含平移和平旋未知数
for (int i = 0; i < n; i++)
{
XYZPoint image = imagePoints[i];
XYZPoint control = controlPoints[i];
// 从相机矩阵中提取必要的部分并填充到系统矩阵
system[i, 0] = cameraMatrix.Row(0)[0];
system[i, 1] = cameraMatrix.Row(1)[0];
system[i, 2] = cameraMatrix.Row(2)[0];
system[i, 3] = -cameraMatrix.Row(3)[0]; // 对应于x分量
system[i, 4] = cameraMatrix.Row(0)[1];
system[i, 5] = cameraMatrix.Row(1)[1];
system[i, 6] = cameraMatrix.Row(2)[1];
system[i, 7] = -cameraMatrix.Row(3)[1]; // 对应于y分量
// 根据像点和控制点坐标计算偏移
unknowns[0] += image.X * trueNorthDirection.Z;
unknowns[1] += image.Y * trueNorthDirection.Z;
unknowns[2] += image.Z;
unknowns[3] += image.X * control.Z - control.X * trueNorthDirection.Z;
unknowns[4] += image.Y * control.Z - control.Y * trueNorthDirection.Z;
unknowns[5] += image.Z * control.Z - control.Z;
}
// 解线性系统求得平移和平旋
var solution = GaussianEliminationSolver.Solve(system, unknowns);
TranslateAndRotate(solution); // 应用解得到的平移和平旋
}
// 实现平移和旋转函数
private void TranslateAndRotate(double[] solution)
{
// ... 这里将solution数组转换为具体的平移和旋转操作
}
}
// 简化的XYZ点结构体
public struct XYZPoint
{
public double X { get; set; }
public double Y { get; set; }
public double Z { get; set; }
}
}
```
阅读全文
相关推荐
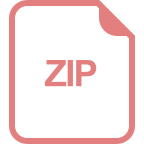
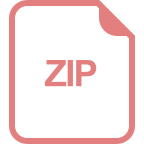
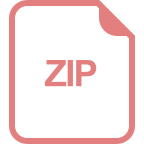
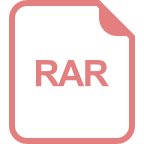
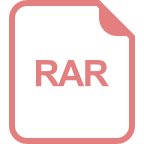
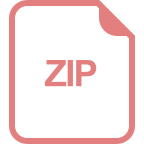
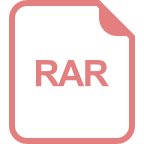
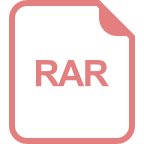
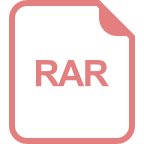
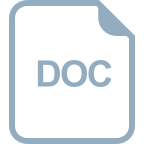




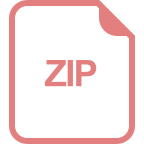
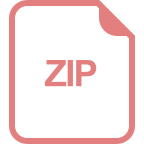