c语言 用顺序表 给定一个整数数组 n和一个目标值 target,请你在该数组中找出和为目标值的那 两个 整数,并返回他们的数组下标。 你可以假设每种输入只会对应一个答案。但是,你不能重复利用这个数组中同一个元素进行求和。
时间: 2024-12-02 15:14:05 浏览: 0
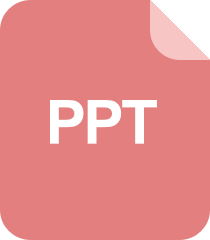
数组应用举例求出十个数中的最大数-C语言数组教学
在 C 语言中,我们可以使用顺序表(也称为数组)来解决这个问题,通过遍历数组并使用哈希表来存储每个遇到过的数字及其索引,以避免重复计算。以下是基本的算法步骤:
1. 初始化两个指针 `left` 和 `right` 分别指向数组的第一个和最后一个元素。
2. 创建一个空的哈希表(如结构体或映射)来存储已访问的数值及其索引。
3. 使用一个 while 循环,当左指针小于右指针时继续:
a. 计算当前 `left` 和 `right` 指向的元素之和。
b. 如果这个和等于目标值 `target`,我们找到了一对和为目标值的元素,返回它们的索引,例如 `(left, right)`。
c. 如果和小于目标值,说明我们需要更大的数,所以将 `left` 指针加一。
d. 否则,如果和大于目标值,说明我们需要更小的数,所以将 `right` 指针减一。
4. 如果循环结束还没有找到解,说明不存在这样的两数之和,返回 NULL 或类似表示未找到的标记。
```c
#include <stdio.h>
#include <stdbool.h>
// 定义结构体表示键值对
typedef struct {
int key;
int index;
} Pair;
// 查找和为目标值的两个元素
int* twoSum(int arr[], int n, int target) {
Pair hash[100]; // 假设最多有100个唯一元素
int left = 0, right = n - 1;
for (int i = 0; i < n; ++i) {
if (hash[i].key == target - arr[i]) { // 已存在目标差值的元素
return &arr[hash[i].index];
}
hash[i].key = arr[i]; // 更新哈希表
hash[i].index = i;
// 探索其他可能的组合
while (left < right && hash[right].key + arr[left] > target) {
right--;
}
if (left < right && hash[right].key + arr[left] == target) {
return &arr[left], &arr[right]; // 返回左右指针指向的元素
} else {
left++;
}
}
return NULL; // 没有找到匹配的两个元素
}
int main() {
int arr[] = {2, 7, 11, 15};
int n = sizeof(arr) / sizeof(arr[0]);
int target = 9;
int* result = twoSum(arr, n, target);
if (result != NULL) {
printf("The two numbers with sum %d are at indices %d and %d.\n", target, result[0], result[1]);
} else {
printf("No pair found with the given target.\n");
}
return 0;
}
```
阅读全文
相关推荐
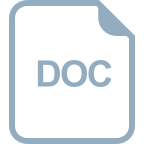
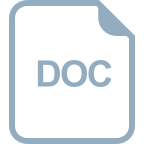













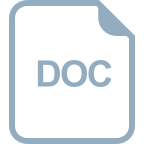
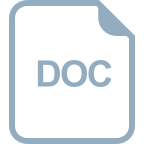
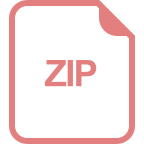