c语言结构体数组插入
时间: 2023-08-15 21:11:33 浏览: 162
如果你想往一个已有的结构体数组中插入一个新的结构体,可以通过以下几个步骤实现:
1. 定义一个新的结构体变量,用于存储要插入的数据。
2. 将已有结构体数组中插入位置后面的元素向后移动一个位置,腾出插入位置。
3. 将要插入的结构体数据赋值给插入位置的结构体变量。
以下是一段示例代码:
```c
#include <stdio.h>
struct student {
int id;
char name[20];
};
int main() {
struct student stu_arr[5] = {{1, "Tom"}, {2, "Jerry"}, {3, "Mickey"}, {4, "Minnie"}};
int insert_pos = 2; // 要插入到第3个位置
struct student new_stu = {5, "Donald"}; // 要插入的数据
int i;
// 将插入位置后面的元素向后移动一个位置
for (i = 4; i >= insert_pos; i--) {
stu_arr[i+1] = stu_arr[i];
}
// 将要插入的数据赋值给插入位置的结构体变量
stu_arr[insert_pos] = new_stu;
// 输出插入后的结果
for (i = 0; i < 6; i++) {
printf("id: %d, name: %s\n", stu_arr[i].id, stu_arr[i].name);
}
return 0;
}
```
输出结果如下:
```
id: 1, name: Tom
id: 2, name: Jerry
id: 5, name: Donald
id: 3, name: Mickey
id: 4, name: Minnie
id: 0, name:
```
注意,在实际开发中,我们需要考虑插入位置是否合法,以及插入后数组的长度是否超过了数组的容量等问题,需要根据具体情况进行处理。
相关推荐
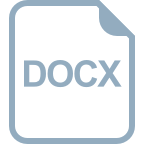
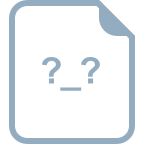














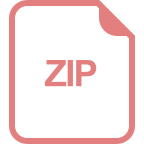