如何将一个文本文件中记录的坐标信息转换为规范化坐标系下的坐标 并且将转化后的坐标进行放大缩小在C++中编写代码实现
时间: 2024-02-12 10:09:43 浏览: 76
以下是一个将文本文件中记录的坐标信息转换为规范化坐标系下的坐标,并对转换后的坐标进行放大缩小的C++代码实现:
```
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
void convertToNormalizedCoordinate(vector<pair<float, float>>& coords, float screenWidth, float screenHeight) {
float xMin = coords[0].first, yMin = coords[0].second;
float xMax = coords[0].first, yMax = coords[0].second;
// Find the minimum and maximum coordinate values
for (int i = 1; i < coords.size(); i++) {
if (coords[i].first < xMin) xMin = coords[i].first;
if (coords[i].first > xMax) xMax = coords[i].first;
if (coords[i].second < yMin) yMin = coords[i].second;
if (coords[i].second > yMax) yMax = coords[i].second;
}
// Convert each coordinate to normalized coordinate
for (int i = 0; i < coords.size(); i++) {
float xNormalized = (2.0 * (coords[i].first - xMin) / screenWidth) - 1.0;
float yNormalized = 1.0 - (2.0 * (coords[i].second - yMin) / screenHeight);
coords[i] = make_pair(xNormalized, yNormalized);
}
}
void scaleCoordinates(vector<pair<float, float>>& coords, float scale) {
// Scale each coordinate by a given scale factor
for (int i = 0; i < coords.size(); i++) {
coords[i].first *= scale;
coords[i].second *= scale;
}
}
int main() {
string filename = "coordinates.txt";
float screenWidth = 800;
float screenHeight = 600;
float scale = 2.0;
// Read the coordinates from file
vector<pair<float, float>> coords;
ifstream in(filename);
float x, y;
while (in >> x >> y) {
coords.push_back(make_pair(x, y));
}
in.close();
// Convert coordinates to normalized coordinates
convertToNormalizedCoordinate(coords, screenWidth, screenHeight);
// Scale the coordinates
scaleCoordinates(coords, scale);
// Print the normalized and scaled coordinates
for (int i = 0; i < coords.size(); i++) {
cout << "(" << coords[i].first << ", " << coords[i].second << ")" << endl;
}
return 0;
}
```
这段代码首先读取了指定文件中的坐标信息,并将其存储在`vector<pair<float, float>>`类型的变量`coords`中。然后调用`convertToNormalizedCoordinate`函数将坐标转换为规范化坐标系下的坐标,再调用`scaleCoordinates`函数对坐标进行放大缩小。最后,打印输出转换后的坐标。在实际使用中,可以根据需要修改输入文件名、屏幕宽度、屏幕高度和缩放因子等参数。
阅读全文
相关推荐













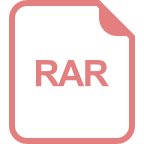

