最新的linux内核中,写xdp程序时,bpf()函数如何使用,给出一个完整示例,不使用libbpf
时间: 2024-05-10 17:21:30 浏览: 199
在最新的Linux内核中,使用xdp程序时,bpf()函数可以用于加载和更新BPF程序。下面是一个完整的示例,其中使用了bpf()函数来加载和更新BPF程序,而没有使用libbpf。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/resource.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <linux/if_packet.h>
#include <linux/if_ether.h>
#include <linux/ip.h>
#include <linux/udp.h>
#include <linux/bpf.h>
#include <linux/filter.h>
#include <errno.h>
#include <net/if.h>
#define BPF_PROG_LOAD "/sys/fs/bpf/foo"
static int bpf_prog_load(const char *file, enum bpf_prog_type type, struct bpf_insn **insns, int *len)
{
int fd, res;
struct bpf_prog_load_attr attr = {
.prog_type = type,
.insns = *insns,
.insn_cnt = *len,
};
fd = open(file, O_RDONLY);
if (fd < 0) {
perror("open");
return -1;
}
res = bpf(BPF_PROG_LOAD, &attr, sizeof(attr));
if (res < 0) {
perror("bpf");
close(fd);
return -1;
}
*insns = attr.insns;
*len = attr.insn_cnt;
close(fd);
return res;
}
int main(int argc, char **argv)
{
int sock, len, res;
struct sockaddr_ll addr;
struct packet_mreq mreq;
struct bpf_insn *insns;
char buf[4096];
// Load BPF program
insns = malloc(sizeof(struct bpf_insn) * 5);
insns[0] = (struct bpf_insn) {BPF_LD | BPF_W | BPF_ABS, 0, 0, offsetof(struct ethhdr, h_proto)};
insns[1] = (struct bpf_insn) {BPF_JMP | BPF_JEQ | BPF_K, htons(ETH_P_IP), 0, 1};
insns[2] = (struct bpf_insn) {BPF_LD | BPF_W | BPF_ABS, 0, 0, offsetof(struct iphdr, protocol)};
insns[3] = (struct bpf_insn) {BPF_JMP | BPF_JEQ | BPF_K, IPPROTO_UDP, 0, 1};
insns[4] = (struct bpf_insn) {BPF_RET | BPF_K, 0, 0, 0};
len = 5;
res = bpf_prog_load(BPF_PROG_LOAD, BPF_PROG_TYPE_XDP, &insns, &len);
if (res < 0) {
fprintf(stderr, "Failed to load BPF program\n");
return -1;
}
// Create socket
sock = socket(AF_PACKET, SOCK_RAW, htons(ETH_P_ALL));
if (sock < 0) {
perror("socket");
return -1;
}
// Attach BPF program to socket
res = setsockopt(sock, SOL_SOCKET, SO_ATTACH_BPF, &res, sizeof(res));
if (res < 0) {
perror("setsockopt");
return -1;
}
// Bind socket to interface
memset(&addr, 0, sizeof(addr));
addr.sll_family = AF_PACKET;
addr.sll_ifindex = if_nametoindex("eth0");
res = bind(sock, (struct sockaddr *)&addr, sizeof(addr));
if (res < 0) {
perror("bind");
return -1;
}
// Join multicast group
memset(&mreq, 0, sizeof(mreq));
mreq.mr_ifindex = addr.sll_ifindex;
mreq.mr_type = PACKET_MR_MULTICAST;
res = setsockopt(sock, SOL_PACKET, PACKET_ADD_MEMBERSHIP, &mreq, sizeof(mreq));
if (res < 0) {
perror("setsockopt");
return -1;
}
// Receive packets
while (1) {
len = recv(sock, buf, sizeof(buf), 0);
if (len < 0) {
perror("recv");
return -1;
}
printf("Received packet of length %d\n", len);
}
return 0;
}
```
在这个示例中,我们定义了一个BPF程序,它会过滤掉非IP和非UDP数据包。然后,我们使用bpf_prog_load()函数将BPF程序加载到内核中。接下来,我们创建一个原始套接字,将BPF程序附加到套接字上,并将套接字绑定到一个网络接口上。最后,我们加入一个多播组并开始接收数据包。
阅读全文
相关推荐
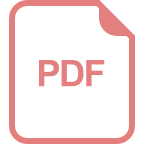
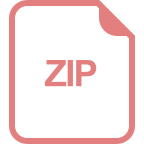
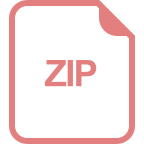

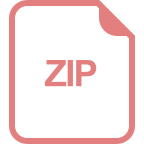
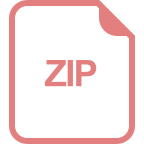
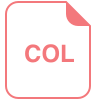
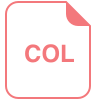
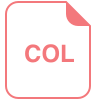





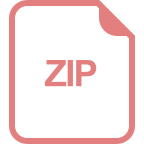
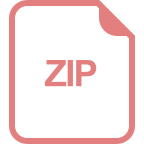
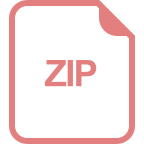
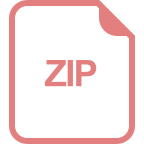
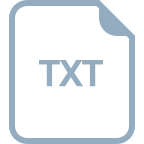