script+js+mysql写购物页面点击按钮加入购物车库
时间: 2023-08-02 14:07:28 浏览: 59
好的,下面是一个简单的购物页面点击按钮加入购物车的示例,使用了JavaScript、MySQL和PHP来实现:
1. 首先,创建一个MySQL数据库,包含两个表:一个用于存储商品信息,另一个用于存储购物车信息。
商品信息表(products)包含以下字段:
- id: 商品ID,自增长整数,作为主键
- name: 商品名称,字符串
- description: 商品描述,字符串
- price: 商品价格,浮点数
购物车信息表(cart)包含以下字段:
- id: 购物车项ID,自增长整数,作为主键
- product_id: 购物车项对应的商品ID,整数
- quantity: 购物车项中商品的数量,整数
2. 在HTML页面中,为每个商品添加一个“加入购物车”按钮,并为按钮添加一个点击事件处理程序。点击按钮时,JavaScript代码会向服务器发送一个HTTP请求,将商品ID添加到购物车表中。
```html
<!DOCTYPE html>
<html>
<head>
<title>购物页面</title>
</head>
<body>
<h1>商品列表</h1>
<ul>
<li>
<h2>商品1</h2>
<p>描述商品1的信息。</p>
<p>价格:$10.00</p>
<button class="add-to-cart" data-product-id="1">加入购物车</button>
</li>
<li>
<h2>商品2</h2>
<p>描述商品2的信息。</p>
<p>价格:$20.00</p>
<button class="add-to-cart" data-product-id="2">加入购物车</button>
</li>
<!-- 添加更多商品... -->
</ul>
<h2>购物车</h2>
<ul id="cart"></ul>
<script>
// 获取所有“加入购物车”按钮
const addToCartButtons = document.querySelectorAll('.add-to-cart');
// 为所有“加入购物车”按钮添加点击事件处理程序
addToCartButtons.forEach(button => {
button.addEventListener('click', function() {
// 获取按钮所对应的商品ID
const productId = button.getAttribute('data-product-id');
// 发送HTTP请求将商品ID添加到购物车表中
const xhr = new XMLHttpRequest();
const url = 'add_to_cart.php';
const params = `product_id=${productId}`;
xhr.open('POST', url, true);
xhr.setRequestHeader('Content-type', 'application/x-www-form-urlencoded');
xhr.onreadystatechange = function() {
if(xhr.readyState == 4 && xhr.status == 200) {
// 更新购物车列表
const cartList = document.getElementById('cart');
cartList.innerHTML = xhr.responseText;
}
};
xhr.send(params);
});
});
</script>
</body>
</html>
```
3. 在服务器端,创建一个PHP脚本(add_to_cart.php),用于将商品ID添加到购物车表中。PHP代码将商品ID插入到购物车表中,并返回最新的购物车列表HTML代码。注意,这是一个简单的示例,实际项目中需要考虑更多的安全性和性能方面的问题。
```php
<?php
// 连接到MySQL数据库
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = "database";
$conn = new mysqli($servername, $username, $password, $dbname);
// 检查连接是否成功
if ($conn->connect_error) {
die("连接失败: " . $conn->connect_error);
}
// 获取要添加到购物车的商品ID
$productId = $_POST['product_id'];
// 将商品ID插入到购物车表中
$sql = "INSERT INTO cart (product_id, quantity) VALUES ($productId, 1)";
if ($conn->query($sql) === FALSE) {
echo "Error: " . $sql . "<br>" . $conn->error;
}
// 获取购物车列表HTML代码并返回
$sql = "SELECT products.name, cart.quantity, products.price FROM cart JOIN products ON cart.product_id=products.id";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
$cartHtml = '';
while($row = $result->fetch_assoc()) {
$cartHtml .= '<li>';
$cartHtml .= '<span>' . $row['name'] . '</span>';
$cartHtml .= '<span>' . $row['quantity'] . '</span>';
$cartHtml .= '<span>' . $row['price'] * $row['quantity'] . '</span>';
$cartHtml .= '</li>';
}
echo $cartHtml;
} else {
echo '购物车为空';
}
// 断开与MySQL数据库的连接
$conn->close();
?>
```
这样,用户在购物页面点击“加入购物车”按钮时,就会向服务器发送一个HTTP请求,将商品ID添加到购物车表中,并将最新的购物车列表HTML代码返回给页面,JavaScript代码会更新购物车列表显示最新的购物车内容。
相关推荐
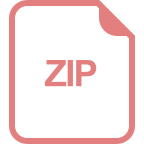
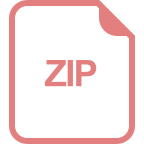
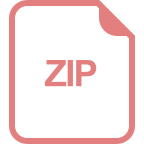














